java.lang.Object
javafx.scene.Node
javafx.scene.Parent
javafx.scene.layout.Region
javafx.scene.control.Control
javafx.scene.control.TextInputControl
javafx.scene.control.TextArea
- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
Text input component that allows a user to enter multiple lines of
plain text. Unlike in previous releases of JavaFX, support for single line
input is not available as part of the TextArea control, however this is
the sole-purpose of the 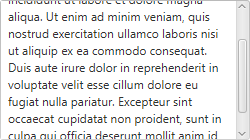
TextField
control. Additionally, if you want
a form of rich-text editing, there is also the
HTMLEditor
control.
TextArea supports the notion of showing prompt text
to the user when there is no text
already in the
TextArea (either via the user, or set programmatically). This is a useful
way of informing the user as to what is expected in the text area, without
having to resort to tooltips
or on-screen labels
.
Example:
var textArea = new TextArea("Lorem ipsum dolor sit amet, consectetur adipiscing elit, "
+ "sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim "
+ "ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip "
+ "ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate "
+ "velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat "
+ "cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.");
textArea.setWrapText(true);
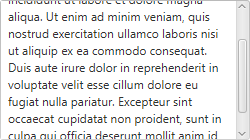
- Since:
- JavaFX 2.0
- See Also:
-
Property Summary
PropertiesTypePropertyDescriptionfinal IntegerProperty
The preferred number of text columns.final IntegerProperty
The preferred number of text rows.final DoubleProperty
The number of pixels by which the content is horizontally scrolled.final DoubleProperty
The number of pixels by which the content is vertically scrolled.final BooleanProperty
If a run of text exceeds the width of theTextArea
, then this variable indicates whether the text should wrap onto another line.Properties declared in class javafx.scene.control.TextInputControl
anchor, caretPosition, editable, font, length, promptText, redoable, selectedText, selection, textFormatter, text, undoable
Properties declared in class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties declared in class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties declared in class javafx.scene.Parent
needsLayout
Properties declared in class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, focusVisible, focusWithin, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Nested Class Summary
Nested classes/interfaces declared in class javafx.scene.control.TextInputControl
TextInputControl.Content
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final int
The default value forprefColumnCount
.static final int
The default value forprefRowCount
.Fields declared in class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields declared in class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionstatic List<CssMetaData<? extends Styleable,
?>> Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.List<CssMetaData<? extends Styleable,
?>> Gets the unmodifiable list of the control's CSS-styleable properties.Returns an unmodifiable list of the character sequences that back the text area's content.final int
Gets the value of theprefColumnCount
property.final int
Gets the value of theprefRowCount
property.final double
Gets the value of thescrollLeft
property.final double
Gets the value of thescrollTop
property.final boolean
Gets the value of thewrapText
property.final IntegerProperty
The preferred number of text columns.final IntegerProperty
The preferred number of text rows.final DoubleProperty
The number of pixels by which the content is horizontally scrolled.final DoubleProperty
The number of pixels by which the content is vertically scrolled.final void
setPrefColumnCount
(int value) Sets the value of theprefColumnCount
property.final void
setPrefRowCount
(int value) Sets the value of theprefRowCount
property.final void
setScrollLeft
(double value) Sets the value of thescrollLeft
property.final void
setScrollTop
(double value) Sets the value of thescrollTop
property.final void
setWrapText
(boolean value) Sets the value of thewrapText
property.final BooleanProperty
If a run of text exceeds the width of theTextArea
, then this variable indicates whether the text should wrap onto another line.Methods declared in class javafx.scene.control.TextInputControl
anchorProperty, appendText, backward, cancelEdit, caretPositionProperty, clear, commitValue, copy, cut, deleteNextChar, deletePreviousChar, deleteText, deleteText, deselect, editableProperty, end, endOfNextWord, extendSelection, fontProperty, forward, getAnchor, getCaretPosition, getContent, getFont, getLength, getPromptText, getSelectedText, getSelection, getText, getText, getTextFormatter, home, insertText, isEditable, isRedoable, isUndoable, lengthProperty, nextWord, paste, positionCaret, previousWord, promptTextProperty, redo, redoableProperty, replaceSelection, replaceText, replaceText, selectAll, selectBackward, selectedTextProperty, selectEnd, selectEndOfNextWord, selectForward, selectHome, selectionProperty, selectNextWord, selectPositionCaret, selectPreviousWord, selectRange, setEditable, setFont, setPromptText, setText, setTextFormatter, textFormatterProperty, textProperty, undo, undoableProperty
Methods declared in class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, contextMenuProperty, createDefaultSkin, getContextMenu, getCssMetaData, getInitialFocusTraversable, getSkin, getTooltip, isResizable, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods declared in class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, computePrefHeight, computePrefWidth, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods declared in class javafx.scene.Parent
getBaselineOffset, getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, layoutChildren, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods declared in class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, focusVisibleProperty, focusWithinProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isFocusVisible, isFocusWithin, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
wrapText
If a run of text exceeds the width of theTextArea
, then this variable indicates whether the text should wrap onto another line. -
prefColumnCount
The preferred number of text columns. This is used for calculating theTextArea
's preferred width. -
prefRowCount
The preferred number of text rows. This is used for calculating theTextArea
's preferred height. -
scrollTop
The number of pixels by which the content is vertically scrolled. -
scrollLeft
The number of pixels by which the content is horizontally scrolled.
-
-
Field Details
-
DEFAULT_PREF_COLUMN_COUNT
public static final int DEFAULT_PREF_COLUMN_COUNTThe default value forprefColumnCount
.- See Also:
-
DEFAULT_PREF_ROW_COUNT
public static final int DEFAULT_PREF_ROW_COUNTThe default value forprefRowCount
.- See Also:
-
-
Constructor Details
-
TextArea
public TextArea()Creates aTextArea
with empty text content. -
TextArea
Creates aTextArea
with initial text content.- Parameters:
text
- A string for text content.
-
-
Method Details
-
getParagraphs
Returns an unmodifiable list of the character sequences that back the text area's content.- Returns:
- an unmodifiable list of the character sequences that back the text area's content
-
wrapTextProperty
If a run of text exceeds the width of theTextArea
, then this variable indicates whether the text should wrap onto another line.- Returns:
- the
wrapText
property - See Also:
-
isWrapText
public final boolean isWrapText()Gets the value of thewrapText
property.- Property description:
- If a run of text exceeds the width of the
TextArea
, then this variable indicates whether the text should wrap onto another line. - Returns:
- the value of the
wrapText
property - See Also:
-
setWrapText
public final void setWrapText(boolean value) Sets the value of thewrapText
property.- Property description:
- If a run of text exceeds the width of the
TextArea
, then this variable indicates whether the text should wrap onto another line. - Parameters:
value
- the value for thewrapText
property- See Also:
-
prefColumnCountProperty
The preferred number of text columns. This is used for calculating theTextArea
's preferred width.- Returns:
- the
prefColumnCount
property - See Also:
-
getPrefColumnCount
public final int getPrefColumnCount()Gets the value of theprefColumnCount
property.- Property description:
- The preferred number of text columns. This is used for
calculating the
TextArea
's preferred width. - Returns:
- the value of the
prefColumnCount
property - See Also:
-
setPrefColumnCount
public final void setPrefColumnCount(int value) Sets the value of theprefColumnCount
property.- Property description:
- The preferred number of text columns. This is used for
calculating the
TextArea
's preferred width. - Parameters:
value
- the value for theprefColumnCount
property- See Also:
-
prefRowCountProperty
The preferred number of text rows. This is used for calculating theTextArea
's preferred height.- Returns:
- the
prefRowCount
property - See Also:
-
getPrefRowCount
public final int getPrefRowCount()Gets the value of theprefRowCount
property.- Property description:
- The preferred number of text rows. This is used for calculating
the
TextArea
's preferred height. - Returns:
- the value of the
prefRowCount
property - See Also:
-
setPrefRowCount
public final void setPrefRowCount(int value) Sets the value of theprefRowCount
property.- Property description:
- The preferred number of text rows. This is used for calculating
the
TextArea
's preferred height. - Parameters:
value
- the value for theprefRowCount
property- See Also:
-
scrollTopProperty
The number of pixels by which the content is vertically scrolled.- Returns:
- the
scrollTop
property - See Also:
-
getScrollTop
public final double getScrollTop()Gets the value of thescrollTop
property.- Property description:
- The number of pixels by which the content is vertically scrolled.
- Returns:
- the value of the
scrollTop
property - See Also:
-
setScrollTop
public final void setScrollTop(double value) Sets the value of thescrollTop
property.- Property description:
- The number of pixels by which the content is vertically scrolled.
- Parameters:
value
- the value for thescrollTop
property- See Also:
-
scrollLeftProperty
The number of pixels by which the content is horizontally scrolled.- Returns:
- the
scrollLeft
property - See Also:
-
getScrollLeft
public final double getScrollLeft()Gets the value of thescrollLeft
property.- Property description:
- The number of pixels by which the content is horizontally scrolled.
- Returns:
- the value of the
scrollLeft
property - See Also:
-
setScrollLeft
public final void setScrollLeft(double value) Sets the value of thescrollLeft
property.- Property description:
- The number of pixels by which the content is horizontally scrolled.
- Parameters:
value
- the value for thescrollLeft
property- See Also:
-
getClassCssMetaData
Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.- Returns:
- the
CssMetaData
- Since:
- JavaFX 8.0
-
getControlCssMetaData
Gets the unmodifiable list of the control's CSS-styleable properties.- Overrides:
getControlCssMetaData
in classTextInputControl
- Returns:
- the unmodifiable list of the control's CSS-styleable properties
- Since:
- JavaFX 8.0
-