- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
A control that has two or more sides, each separated by a divider, which can be dragged by the user to give more space to one of the sides, resulting in the other side shrinking by an equal amount.
Nodes
can be positioned horizontally next to each other, or stacked
vertically. This can be controlled by setting the orientationProperty()
.
The dividers in a SplitPane have the following behavior
- Dividers cannot overlap another divider
- Dividers cannot overlap a node.
- Dividers moving to the left/top will stop when the node's min size is reached.
- Dividers moving to the right/bottom will stop when the node's max size is reached.
Nodes needs to be placed inside a layout container before they are added into the SplitPane. If the node is not inside a layout container the maximum and minimum position of the divider will be the maximum and minimum size of the content.
A divider's position ranges from 0 to 1.0(inclusive). A position of 0 will place the
divider at the left/top most edge of the SplitPane plus the minimum size of the node. A
position of 1.0 will place the divider at the right/bottom most edge of the SplitPane minus the
minimum size of the node. A divider position of 0.5 will place the
the divider in the middle of the SplitPane. Setting the divider position greater
than the node's maximum size position will result in the divider being set at the
node's maximum size position. Setting the divider position less than the node's minimum size position
will result in the divider being set at the node's minimum size position. Therefore the value set in
setDividerPosition(int, double)
and setDividerPositions(double...)
may not be the same as the value returned by
getDividerPositions()
.
If there are more than two nodes in the SplitPane and the divider positions are set in such a way that the dividers cannot fit the nodes the dividers will be automatically adjusted by the SplitPane.
For example we have three nodes whose sizes and divider positions are
Node 1: min 25 max 100 Node 2: min 100 max 200 Node 3: min 25 max 50 divider 1: 0.40 divider 2: 0.45
The result will be Node 1 size will be its pref size and divider 1 will be positioned 0.40, Node 2 size will be its min size and divider 2 position will be the min size of Node 2 plus divider 1 position, and the remaining space will be given to Node 3.
SplitPane sets focusTraversable to false.
Example:
SplitPane sp = new SplitPane();
final StackPane sp1 = new StackPane();
sp1.getChildren().add(new Button("Button One"));
final StackPane sp2 = new StackPane();
sp2.getChildren().add(new Button("Button Two"));
final StackPane sp3 = new StackPane();
sp3.getChildren().add(new Button("Button Three"));
sp.getItems().addAll(sp1, sp2, sp3);
sp.setDividerPositions(0.3f, 0.6f, 0.9f);
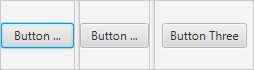
- Since:
- JavaFX 2.0
-
Property Summary
PropertiesProperties declared in class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties declared in class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties declared in class javafx.scene.Parent
needsLayout
Properties declared in class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, focusVisible, focusWithin, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic class
Represents a single divider in the SplitPane. -
Field Summary
Fields declared in class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields declared in class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionstatic List<CssMetaData<? extends Styleable,
?>> Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.List<CssMetaData<? extends Styleable,
?>> Gets the unmodifiable list of the control's CSS-styleable properties.double[]
Returns an array of double containing the position of each divider.Returns an unmodifiable list of all the dividers in this SplitPane.protected Boolean
Returns the initial focus traversable state of this control, for use by the JavaFX CSS engine to correctly set its initial value.getItems()
Returns an ObservableList which can be use to modify the contents of the SplitPane.final Orientation
The orientation for the SplitPane.static Boolean
isResizableWithParent
(Node node) Return true if the node is resizable when the parent container is resized false otherwise.final ObjectProperty<Orientation>
The orientation for the SplitPane.void
setDividerPosition
(int dividerIndex, double position) Sets the position of the divider at the specified divider index.void
setDividerPositions
(double... positions) Sets the position of the dividerfinal void
setOrientation
(Orientation value) This property controls how the SplitPane should be displayed to the user.static void
setResizableWithParent
(Node node, Boolean value) Sets a node in the SplitPane to be resizable or not when the SplitPane is resized.Methods declared in class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, contextMenuProperty, createDefaultSkin, getContextMenu, getCssMetaData, getSkin, getTooltip, isResizable, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods declared in class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, computePrefHeight, computePrefWidth, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods declared in class javafx.scene.Parent
getBaselineOffset, getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, layoutChildren, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods declared in class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, focusVisibleProperty, focusWithinProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isFocusVisible, isFocusWithin, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
orientation
The orientation for the SplitPane.
-
-
Constructor Details
-
SplitPane
public SplitPane()Creates a new SplitPane with no content. -
SplitPane
Creates a new SplitPane with the given items set as the content to split between one or more dividers.- Parameters:
items
- The items to place inside the SplitPane.- Since:
- JavaFX 8u40
-
-
Method Details
-
setResizableWithParent
Sets a node in the SplitPane to be resizable or not when the SplitPane is resized. By default all node are resizable. Setting value to false will prevent the node from being resized.- Parameters:
node
- A node in the SplitPane.value
- true if the node is resizable or false if not resizable.- Since:
- JavaFX 2.1
-
isResizableWithParent
Return true if the node is resizable when the parent container is resized false otherwise.- Default value:
- true
- Parameters:
node
- A node in the SplitPane.- Returns:
- true if the node is resizable false otherwise.
- Since:
- JavaFX 2.1
-
setOrientation
This property controls how the SplitPane should be displayed to the user.
Orientation.HORIZONTAL
will result in two (or more) nodes being placed next to each other horizontally, whilstOrientation.VERTICAL
will result in the nodes being stacked vertically.- Parameters:
value
- the orientation value
-
getOrientation
The orientation for the SplitPane.- Returns:
- The orientation for the SplitPane.
-
orientationProperty
The orientation for the SplitPane.- Returns:
- the orientation property for the SplitPane
- See Also:
-
getItems
Returns an ObservableList which can be use to modify the contents of the SplitPane. The order the nodes are placed into this list will be the same order in the SplitPane.- Returns:
- the list of items in this SplitPane.
-
getDividers
Returns an unmodifiable list of all the dividers in this SplitPane.- Returns:
- the list of dividers.
-
setDividerPosition
public void setDividerPosition(int dividerIndex, double position) Sets the position of the divider at the specified divider index.- Parameters:
dividerIndex
- the index of the divider.position
- the divider position, between 0.0 and 1.0 (inclusive).
-
setDividerPositions
public void setDividerPositions(double... positions) Sets the position of the divider- Parameters:
positions
- the divider position, between 0.0 and 1.0 (inclusive).
-
getDividerPositions
public double[] getDividerPositions()Returns an array of double containing the position of each divider.- Returns:
- an array of double containing the position of each divider.
-
getClassCssMetaData
Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.- Returns:
- the
CssMetaData
- Since:
- JavaFX 8.0
-
getControlCssMetaData
Gets the unmodifiable list of the control's CSS-styleable properties.- Overrides:
getControlCssMetaData
in classControl
- Returns:
- the unmodifiable list of the control's CSS-styleable properties
- Since:
- JavaFX 8.0
-
getInitialFocusTraversable
Returns the initial focus traversable state of this control, for use by the JavaFX CSS engine to correctly set its initial value. This method is overridden as by default UI controls have focus traversable set to true, but that is not appropriate for this control.- Overrides:
getInitialFocusTraversable
in classControl
- Returns:
- the initial focus traversable state of this control
- Since:
- 9
-