- Type Parameters:
S
- The type of the objects contained within the TableView items list.
- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
@DefaultProperty("items") public class TableView<S> extends Control
ListView
control, with the addition of support for columns. For an
example on how to create a TableView, refer to the 'Creating a TableView'
control section below.
The TableView control has a number of features, including:
- Powerful
TableColumn
API:- Support for
cell factories
to easily customizecell
contents in both rendering and editing states. - Specification of
minWidth
/prefWidth
/maxWidth
, and alsofixed width columns
. - Width resizing by the user at runtime.
- Column reordering by the user at runtime.
- Built-in support for
column nesting
- Support for
- Different
resizing policies
to dictate what happens when the user resizes columns. - Support for
multiple column sorting
by clicking the column header (hold down Shift keyboard key whilst clicking on a header to sort by multiple columns).
Note that TableView is intended to be used to visualize data - it is not
intended to be used for laying out your user interface. If you want to lay
your user interface out in a grid-like fashion, consider the
GridPane
layout instead.
Creating a TableView
Creating a TableView is a multi-step process, and also depends on the
underlying data model needing to be represented. For this example we'll use
an ObservableList<Person>, as it is the simplest way of showing data in a
TableView. The Person
class will consist of a first
name and last name properties. That is:
public class Person {
private StringProperty firstName;
public void setFirstName(String value) { firstNameProperty().set(value); }
public String getFirstName() { return firstNameProperty().get(); }
public StringProperty firstNameProperty() {
if (firstName == null) firstName = new SimpleStringProperty(this, "firstName");
return firstName;
}
private StringProperty lastName;
public void setLastName(String value) { lastNameProperty().set(value); }
public String getLastName() { return lastNameProperty().get(); }
public StringProperty lastNameProperty() {
if (lastName == null) lastName = new SimpleStringProperty(this, "lastName");
return lastName;
}
public Person(String firstName, String lastName) {
setFirstName(firstName);
setLastName(lastName);
}
}
The data we will use for this example is:
List<Person> members = List.of(
new Person("William", "Reed"),
new Person("James", "Michaelson"),
new Person("Julius", "Dean"));
Firstly, we need to create a data model. As mentioned, for this example, we'll be using an ObservableList<Person>:
ObservableList<Person> teamMembers = FXCollections.observableArrayList(members);
Then we create a TableView instance:
TableView<Person> table = new TableView<>();
table.setItems(teamMembers);
With the items set as such, TableView will automatically update whenever
the teamMembers
list changes. If the items list is available
before the TableView is instantiated, it is possible to pass it directly into
the constructor:
TableView<Person> table = new TableView<>(teamMembers);
At this point we now have a TableView hooked up to observe the
teamMembers
observableList. The missing ingredient
now is the means of splitting out the data contained within the model and
representing it in one or more TableColumn
instances. To
create a two-column TableView to show the firstName and lastName properties,
we extend the last code sample as follows:
TableColumn<Person, String> firstNameCol = new TableColumn<>("First Name");
firstNameCol.setCellValueFactory(new PropertyValueFactory<>(members.get(0).firstNameProperty().getName())));
TableColumn<Person, String> lastNameCol = new TableColumn<>("Last Name");
lastNameCol.setCellValueFactory(new PropertyValueFactory<>(members.get(0).lastNameProperty().getName())));
table.getColumns().setAll(firstNameCol, lastNameCol);
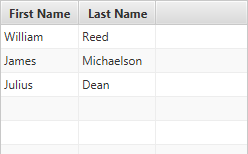
With the code shown above we have fully defined the minimum properties required to create a TableView instance. Running this code will result in the TableView being shown with two columns for firstName and lastName. Any other properties of the Person class will not be shown, as no TableColumns are defined.
TableView support for classes that don't contain properties
The code shown above is the shortest possible code for creating a TableView
when the domain objects are designed with JavaFX properties in mind
(additionally, PropertyValueFactory
supports
normal JavaBean properties too, although there is a caveat to this, so refer
to the class documentation for more information). When this is not the case,
it is necessary to provide a custom cell value factory. More information
about cell value factories can be found in the TableColumn
API
documentation, but briefly, here is how a TableColumn could be specified:
firstNameCol.setCellValueFactory(new Callback<CellDataFeatures<Person, String>, ObservableValue<String>>() {
public ObservableValue<String> call(CellDataFeatures<Person, String> p) {
// p.getValue() returns the Person instance for a particular TableView row
return p.getValue().firstNameProperty();
}
});
// or with a lambda expression:
firstNameCol.setCellValueFactory(p -> p.getValue().firstNameProperty());
TableView Selection / Focus APIs
To track selection and focus, it is necessary to become familiar with the
SelectionModel
and FocusModel
classes. A TableView has at most
one instance of each of these classes, available from
selectionModel
and
focusModel
properties respectively.
Whilst it is possible to use this API to set a new selection model, in
most circumstances this is not necessary - the default selection and focus
models should work in most circumstances.
The default SelectionModel
used when instantiating a TableView is
an implementation of the MultipleSelectionModel
abstract class.
However, as noted in the API documentation for
the selectionMode
property, the default value is SelectionMode.SINGLE
. To enable
multiple selection in a default TableView instance, it is therefore necessary
to do the following:
tableView.getSelectionModel().setSelectionMode(SelectionMode.MULTIPLE);
Customizing TableView Visuals
The visuals of the TableView can be entirely customized by replacing the
default row factory
. A row factory is used to
generate TableRow
instances, which are used to represent an entire
row in the TableView.
In many cases, this is not what is desired however, as it is more commonly
the case that cells be customized on a per-column basis, not a per-row basis.
It is therefore important to note that a TableRow
is not a
TableCell
. A TableRow
is simply a container for zero or more
TableCell
, and in most circumstances it is more likely that you'll
want to create custom TableCells, rather than TableRows. The primary use case
for creating custom TableRow instances would most probably be to introduce
some form of column spanning support.
You can create custom TableCell
instances per column by assigning
the appropriate function to the TableColumn
cell factory
property.
See the Cell
class documentation for a more complete
description of how to write custom Cells.
Sorting
Prior to JavaFX 8.0, the TableView control would treat the
items
list as the view model, meaning that any changes to
the list would be immediately reflected visually. TableView would also modify
the order of this list directly when a user initiated a sort. This meant that
(again, prior to JavaFX 8.0) it was not possible to have the TableView return
to an unsorted state (after iterating through ascending and descending
orders).
Starting with JavaFX 8.0 (and the introduction of SortedList
), it
is now possible to have the collection return to the unsorted state when
there are no columns as part of the TableView
sort order
. To do this, you must create a SortedList
instance, and bind its
comparator
property to the TableView comparator
property,
list so:
// create a SortedList based on the provided ObservableList
SortedList sortedList = new SortedList(FXCollections.observableArrayList(2, 1, 3));
// create a TableView with the sorted list set as the items it will show
final TableView<Integer> tableView = new TableView<>(sortedList);
// bind the sortedList comparator to the TableView comparator
sortedList.comparatorProperty().bind(tableView.comparatorProperty());
// Don't forget to define columns!
Editing
This control supports inline editing of values, and this section attempts to give an overview of the available APIs and how you should use them.
Firstly, cell editing most commonly requires a different user interface
than when a cell is not being edited. This is the responsibility of the
Cell
implementation being used. For TableView, it is highly
recommended that editing be
per-TableColumn
,
rather than per row
, as more often than not
you want users to edit each column value differently, and this approach allows
for editors specific to each column. It is your choice whether the cell is
permanently in an editing state (e.g. this is common for CheckBox
cells),
or to switch to a different UI when editing begins (e.g. when a double-click
is received on a cell).
To know when editing has been requested on a cell,
simply override the Cell.startEdit()
method, and
update the cell text
and
graphic
properties as
appropriate (e.g. set the text to null and set the graphic to be a
TextField
). Additionally, you should also override
Cell.cancelEdit()
to reset the UI back to its original visual state
when the editing concludes. In both cases it is important that you also
ensure that you call the super method to have the cell perform all duties it
must do to enter or exit its editing mode.
Once your cell is in an editing state, the next thing you are most probably
interested in is how to commit or cancel the editing that is taking place. This is your
responsibility as the cell factory provider. Your cell implementation will know
when the editing is over, based on the user input (e.g. when the user presses
the Enter or ESC keys on their keyboard). When this happens, it is your
responsibility to call Cell.commitEdit(Object)
or
Cell.cancelEdit()
, as appropriate.
When you call Cell.commitEdit(Object)
an event is fired to the
TableView, which you can observe by adding an EventHandler
via
TableColumn.setOnEditCommit(javafx.event.EventHandler)
. Similarly,
you can also observe edit events for
edit start
and edit cancel
.
By default the TableColumn edit commit handler is non-null, with a default
handler that attempts to overwrite the property value for the
item in the currently-being-edited row. It is able to do this as the
Cell.commitEdit(Object)
method is passed in the new value, and this
is passed along to the edit commit handler via the
CellEditEvent
that is
fired. It is simply a matter of calling
TableColumn.CellEditEvent.getNewValue()
to
retrieve this value.
It is very important to note that if you call
TableColumn.setOnEditCommit(javafx.event.EventHandler)
with your own
EventHandler
, then you will be removing the default handler. Unless
you then handle the writeback to the property (or the relevant data source),
nothing will happen. You can work around this by using the
TableColumnBase.addEventHandler(javafx.event.EventType, javafx.event.EventHandler)
method to add a TableColumn.editCommitEvent()
EventType
with
your desired EventHandler
as the second argument. Using this method,
you will not replace the default implementation, but you will be notified when
an edit commit has occurred.
Hopefully this summary answers some of the commonly asked questions. Fortunately, JavaFX ships with a number of pre-built cell factories that handle all the editing requirements on your behalf. You can find these pre-built cell factories in the javafx.scene.control.cell package.
- Since:
- JavaFX 2.0
- See Also:
TableColumn
,TablePosition
-
Property Summary
Properties Type Property Description ObjectProperty<Callback<TableView.ResizeFeatures,Boolean>>
columnResizePolicy
Called when the user completes a column-resize operation.ReadOnlyObjectProperty<Comparator<S>>
comparator
The comparator property is a read-only property that is representative of the current state of thesort order
list.BooleanProperty
editable
Specifies whether this TableView is editable - only if the TableView, the TableColumn (if applicable) and the TableCells within it are both editable will a TableCell be able to go into their editing state.ReadOnlyObjectProperty<TablePosition<S,?>>
editingCell
Represents the current cell being edited, or null if there is no cell being edited.DoubleProperty
fixedCellSize
Specifies whether this control has cells that are a fixed height (of the specified value).ObjectProperty<TableView.TableViewFocusModel<S>>
focusModel
Represents the currently-installedTableView.TableViewFocusModel
for this TableView.ObjectProperty<ObservableList<S>>
items
The underlying data model for the TableView.ObjectProperty<EventHandler<ScrollToEvent<TableColumn<S,?>>>>
onScrollToColumn
Called when there's a request to scroll a column into view usingscrollToColumn(TableColumn)
orscrollToColumnIndex(int)
ObjectProperty<EventHandler<ScrollToEvent<Integer>>>
onScrollTo
Called when there's a request to scroll an index into view usingscrollTo(int)
orscrollTo(Object)
ObjectProperty<EventHandler<SortEvent<TableView<S>>>>
onSort
Called when there's a request to sort the control.ObjectProperty<Node>
placeholder
This Node is shown to the user when the table has no content to show.ObjectProperty<Callback<TableView<S>,TableRow<S>>>
rowFactory
A function which produces a TableRow.ObjectProperty<TableView.TableViewSelectionModel<S>>
selectionModel
The SelectionModel provides the API through which it is possible to select single or multiple items within a TableView, as well as inspect which items have been selected by the user.ObjectProperty<Callback<TableView<S>,Boolean>>
sortPolicy
The sort policy specifies how sorting in this TableView should be performed.BooleanProperty
tableMenuButtonVisible
This controls whether a menu button is available when the user clicks in a designated space within the TableView, within which is a radio menu item for each TableColumn in this table.Properties inherited from class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties inherited from class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Nested Class Summary
Nested Classes Modifier and Type Class Description static class
TableView.ResizeFeatures<S>
An immutable wrapper class for use in the TableViewcolumn resize
functionality.static class
TableView.TableViewFocusModel<S>
AFocusModel
with additional functionality to support the requirements of a TableView control.static class
TableView.TableViewSelectionModel<S>
A simple extension of theSelectionModel
abstract class to allow for special support for TableView controls. -
Field Summary
Fields Modifier and Type Field Description static Callback<TableView.ResizeFeatures,Boolean>
CONSTRAINED_RESIZE_POLICY
Simple policy that ensures the width of all visible leaf columns in this table sum up to equal the width of the table itself.static Callback<TableView,Boolean>
DEFAULT_SORT_POLICY
The defaultsort policy
that this TableView will use if no other policy is specified.static Callback<TableView.ResizeFeatures,Boolean>
UNCONSTRAINED_RESIZE_POLICY
Very simple resize policy that just resizes the specified column by the provided delta and shifts all other columns (to the right of the given column) further to the right (when the delta is positive) or to the left (when the delta is negative). -
Constructor Summary
Constructors Constructor Description TableView()
Creates a default TableView control with no content.TableView(ObservableList<S> items)
Creates a TableView with the content provided in the items ObservableList. -
Method Summary
Modifier and Type Method Description ObjectProperty<Callback<TableView.ResizeFeatures,Boolean>>
columnResizePolicyProperty()
Called when the user completes a column-resize operation.ReadOnlyObjectProperty<Comparator<S>>
comparatorProperty()
The comparator property is a read-only property that is representative of the current state of thesort order
list.protected Skin<?>
createDefaultSkin()
Create a new instance of the default skin for this control.void
edit(int row, TableColumn<S,?> column)
Causes the cell at the given row/column view indexes to switch into its editing state, if it is not already in it, and assuming that the TableView and column are also editable.BooleanProperty
editableProperty()
Specifies whether this TableView is editable - only if the TableView, the TableColumn (if applicable) and the TableCells within it are both editable will a TableCell be able to go into their editing state.ReadOnlyObjectProperty<TablePosition<S,?>>
editingCellProperty()
Represents the current cell being edited, or null if there is no cell being edited.DoubleProperty
fixedCellSizeProperty()
Specifies whether this control has cells that are a fixed height (of the specified value).ObjectProperty<TableView.TableViewFocusModel<S>>
focusModelProperty()
Represents the currently-installedTableView.TableViewFocusModel
for this TableView.static List<CssMetaData<? extends Styleable,?>>
getClassCssMetaData()
Callback<TableView.ResizeFeatures,Boolean>
getColumnResizePolicy()
Gets the value of the property columnResizePolicy.ObservableList<TableColumn<S,?>>
getColumns()
The TableColumns that are part of this TableView.Comparator<S>
getComparator()
Gets the value of the property comparator.List<CssMetaData<? extends Styleable,?>>
getControlCssMetaData()
TablePosition<S,?>
getEditingCell()
Gets the value of the property editingCell.double
getFixedCellSize()
Returns the fixed cell size value.TableView.TableViewFocusModel<S>
getFocusModel()
Gets the value of the property focusModel.ObservableList<S>
getItems()
Gets the value of the property items.EventHandler<ScrollToEvent<Integer>>
getOnScrollTo()
Gets the value of the property onScrollTo.EventHandler<ScrollToEvent<TableColumn<S,?>>>
getOnScrollToColumn()
Gets the value of the property onScrollToColumn.EventHandler<SortEvent<TableView<S>>>
getOnSort()
Gets the value of the property onSort.Node
getPlaceholder()
Gets the value of the property placeholder.Callback<TableView<S>,TableRow<S>>
getRowFactory()
Gets the value of the property rowFactory.TableView.TableViewSelectionModel<S>
getSelectionModel()
Gets the value of the property selectionModel.ObservableList<TableColumn<S,?>>
getSortOrder()
The sortOrder list defines the order in whichTableColumn
instances are sorted.Callback<TableView<S>,Boolean>
getSortPolicy()
Gets the value of the property sortPolicy.TableColumn<S,?>
getVisibleLeafColumn(int column)
Returns the TableColumn in the given column index, relative to all other visible leaf columns.ObservableList<TableColumn<S,?>>
getVisibleLeafColumns()
Returns an unmodifiable list containing the currently visible leaf columns.int
getVisibleLeafIndex(TableColumn<S,?> column)
Returns the position of the given column, relative to all other visible leaf columns.boolean
isEditable()
Gets the value of the property editable.boolean
isTableMenuButtonVisible()
Gets the value of the property tableMenuButtonVisible.ObjectProperty<ObservableList<S>>
itemsProperty()
The underlying data model for the TableView.ObjectProperty<EventHandler<ScrollToEvent<TableColumn<S,?>>>>
onScrollToColumnProperty()
Called when there's a request to scroll a column into view usingscrollToColumn(TableColumn)
orscrollToColumnIndex(int)
ObjectProperty<EventHandler<ScrollToEvent<Integer>>>
onScrollToProperty()
Called when there's a request to scroll an index into view usingscrollTo(int)
orscrollTo(Object)
ObjectProperty<EventHandler<SortEvent<TableView<S>>>>
onSortProperty()
Called when there's a request to sort the control.ObjectProperty<Node>
placeholderProperty()
This Node is shown to the user when the table has no content to show.Object
queryAccessibleAttribute(AccessibleAttribute attribute, Object... parameters)
This method is called by the assistive technology to request the value for an attribute.void
refresh()
Callingrefresh()
forces the TableView control to recreate and repopulate the cells necessary to populate the visual bounds of the control.boolean
resizeColumn(TableColumn<S,?> column, double delta)
Applies the currently installed resize policy against the given column, resizing it based on the delta value provided.ObjectProperty<Callback<TableView<S>,TableRow<S>>>
rowFactoryProperty()
A function which produces a TableRow.void
scrollTo(int index)
Scrolls the TableView so that the given index is visible within the viewport.void
scrollTo(S object)
Scrolls the TableView so that the given object is visible within the viewport.void
scrollToColumn(TableColumn<S,?> column)
Scrolls the TableView so that the given column is visible within the viewport.void
scrollToColumnIndex(int columnIndex)
Scrolls the TableView so that the given index is visible within the viewport.ObjectProperty<TableView.TableViewSelectionModel<S>>
selectionModelProperty()
The SelectionModel provides the API through which it is possible to select single or multiple items within a TableView, as well as inspect which items have been selected by the user.void
setColumnResizePolicy(Callback<TableView.ResizeFeatures,Boolean> callback)
Sets the value of the property columnResizePolicy.void
setEditable(boolean value)
Sets the value of the property editable.void
setFixedCellSize(double value)
Sets the new fixed cell size for this control.void
setFocusModel(TableView.TableViewFocusModel<S> value)
Sets the value of the property focusModel.void
setItems(ObservableList<S> value)
Sets the value of the property items.void
setOnScrollTo(EventHandler<ScrollToEvent<Integer>> value)
Sets the value of the property onScrollTo.void
setOnScrollToColumn(EventHandler<ScrollToEvent<TableColumn<S,?>>> value)
Sets the value of the property onScrollToColumn.void
setOnSort(EventHandler<SortEvent<TableView<S>>> value)
Sets the value of the property onSort.void
setPlaceholder(Node value)
Sets the value of the property placeholder.void
setRowFactory(Callback<TableView<S>,TableRow<S>> value)
Sets the value of the property rowFactory.void
setSelectionModel(TableView.TableViewSelectionModel<S> value)
Sets the value of the property selectionModel.void
setSortPolicy(Callback<TableView<S>,Boolean> callback)
Sets the value of the property sortPolicy.void
setTableMenuButtonVisible(boolean value)
Sets the value of the property tableMenuButtonVisible.void
sort()
The sort method forces the TableView to re-run its sorting algorithm.ObjectProperty<Callback<TableView<S>,Boolean>>
sortPolicyProperty()
The sort policy specifies how sorting in this TableView should be performed.BooleanProperty
tableMenuButtonVisibleProperty()
This controls whether a menu button is available when the user clicks in a designated space within the TableView, within which is a radio menu item for each TableColumn in this table.Methods inherited from class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, computePrefHeight, computePrefWidth, contextMenuProperty, executeAccessibleAction, getBaselineOffset, getContextMenu, getCssMetaData, getInitialFocusTraversable, getSkin, getTooltip, isResizable, layoutChildren, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods inherited from class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods inherited from class javafx.scene.Parent
getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, lookup, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods inherited from class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, fireEvent, focusedProperty, focusTraversableProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
-
Property Details
-
items
The underlying data model for the TableView. Note that it has a generic type that must match the type of the TableView itself.- See Also:
getItems()
,setItems(ObservableList)
-
tableMenuButtonVisible
This controls whether a menu button is available when the user clicks in a designated space within the TableView, within which is a radio menu item for each TableColumn in this table. This menu allows for the user to show and hide all TableColumns easily. -
columnResizePolicy
Called when the user completes a column-resize operation. The two most common policies are available as static functions in the TableView class:UNCONSTRAINED_RESIZE_POLICY
andCONSTRAINED_RESIZE_POLICY
. -
rowFactory
A function which produces a TableRow. The system is responsible for reusing TableRows. Return from this function a TableRow which might be usable for representing a single row in a TableView.Note that a TableRow is not a TableCell. A TableRow is simply a container for a TableCell, and in most circumstances it is more likely that you'll want to create custom TableCells, rather than TableRows. The primary use case for creating custom TableRow instances would most probably be to introduce some form of column spanning support.
You can create custom TableCell instances per column by assigning the appropriate function to the cellFactory property in the TableColumn class.
- See Also:
getRowFactory()
,setRowFactory(Callback)
-
placeholder
This Node is shown to the user when the table has no content to show. This may be the case because the table model has no data in the first place, that a filter has been applied to the table model, resulting in there being nothing to show the user, or that there are no currently visible columns.- See Also:
getPlaceholder()
,setPlaceholder(Node)
-
selectionModel
The SelectionModel provides the API through which it is possible to select single or multiple items within a TableView, as well as inspect which items have been selected by the user. Note that it has a generic type that must match the type of the TableView itself. -
focusModel
Represents the currently-installedTableView.TableViewFocusModel
for this TableView. Under almost all circumstances leaving this as the default focus model will suffice. -
editable
Specifies whether this TableView is editable - only if the TableView, the TableColumn (if applicable) and the TableCells within it are both editable will a TableCell be able to go into their editing state.- See Also:
isEditable()
,setEditable(boolean)
-
fixedCellSize
Specifies whether this control has cells that are a fixed height (of the specified value). If this value is less than or equal to zero, then all cells are individually sized and positioned. This is a slow operation. Therefore, when performance matters and developers are not dependent on variable cell sizes it is a good idea to set the fixed cell size value. Generally cells are around 24px, so setting a fixed cell size of 24 is likely to result in very little difference in visuals, but a improvement to performance.To set this property via CSS, use the -fx-fixed-cell-size property. This should not be confused with the -fx-cell-size property. The difference between these two CSS properties is that -fx-cell-size will size all cells to the specified size, but it will not enforce that this is the only size (thus allowing for variable cell sizes, and preventing the performance gains from being possible). Therefore, when performance matters use -fx-fixed-cell-size, instead of -fx-cell-size. If both properties are specified in CSS, -fx-fixed-cell-size takes precedence.
- Since:
- JavaFX 8.0
- See Also:
getFixedCellSize()
,setFixedCellSize(double)
-
editingCell
Represents the current cell being edited, or null if there is no cell being edited.- See Also:
getEditingCell()
-
comparator
The comparator property is a read-only property that is representative of the current state of thesort order
list. The sort order list contains the columns that have been added to it either programmatically or via a user clicking on the headers themselves.- Since:
- JavaFX 8.0
- See Also:
getComparator()
-
sortPolicy
The sort policy specifies how sorting in this TableView should be performed. For example, a basic sort policy may just callFXCollections.sort(tableView.getItems())
, whereas a more advanced sort policy may call to a database to perform the necessary sorting on the server-side.TableView ships with a
default sort policy
that does precisely as mentioned above: it simply attempts to sort the items list in-place.It is recommended that rather than override the
sort
method that a different sort policy be provided instead.- Since:
- JavaFX 8.0
- See Also:
getSortPolicy()
,setSortPolicy(Callback)
-
onSort
Called when there's a request to sort the control.- Since:
- JavaFX 8.0
- See Also:
getOnSort()
,setOnSort(EventHandler)
-
onScrollTo
Called when there's a request to scroll an index into view usingscrollTo(int)
orscrollTo(Object)
- Since:
- JavaFX 8.0
- See Also:
getOnScrollTo()
,setOnScrollTo(EventHandler)
-
onScrollToColumn
Called when there's a request to scroll a column into view usingscrollToColumn(TableColumn)
orscrollToColumnIndex(int)
- Since:
- JavaFX 8.0
- See Also:
getOnScrollToColumn()
,setOnScrollToColumn(EventHandler)
-
-
Field Details
-
UNCONSTRAINED_RESIZE_POLICY
Very simple resize policy that just resizes the specified column by the provided delta and shifts all other columns (to the right of the given column) further to the right (when the delta is positive) or to the left (when the delta is negative).
It also handles the case where we have nested columns by sharing the new space, or subtracting the removed space, evenly between all immediate children columns. Of course, the immediate children may themselves be nested, and they would then use this policy on their children.
-
CONSTRAINED_RESIZE_POLICY
Simple policy that ensures the width of all visible leaf columns in this table sum up to equal the width of the table itself.
When the user resizes a column width with this policy, the table automatically adjusts the width of the right hand side columns. When the user increases a column width, the table decreases the width of the rightmost column until it reaches its minimum width. Then it decreases the width of the second rightmost column until it reaches minimum width and so on. When all right hand side columns reach minimum size, the user cannot increase the size of resized column any more.
-
DEFAULT_SORT_POLICY
The defaultsort policy
that this TableView will use if no other policy is specified. The sort policy is a simpleCallback
that accepts a TableView as the sole argument and expects a Boolean response representing whether the sort succeeded or not. A Boolean response of true represents success, and a response of false (or null) will be considered to represent failure.- Since:
- JavaFX 8.0
-
-
Constructor Details
-
TableView
public TableView()Creates a default TableView control with no content.Refer to the
TableView
class documentation for details on the default state of other properties. -
TableView
Creates a TableView with the content provided in the items ObservableList. This also sets up an observer such that any changes to the items list will be immediately reflected in the TableView itself.Refer to the
TableView
class documentation for details on the default state of other properties.- Parameters:
items
- The items to insert into the TableView, and the list to watch for changes (to automatically show in the TableView).
-
-
Method Details
-
itemsProperty
The underlying data model for the TableView. Note that it has a generic type that must match the type of the TableView itself.- See Also:
getItems()
,setItems(ObservableList)
-
setItems
Sets the value of the property items.- Property description:
- The underlying data model for the TableView. Note that it has a generic type that must match the type of the TableView itself.
-
getItems
Gets the value of the property items.- Property description:
- The underlying data model for the TableView. Note that it has a generic type that must match the type of the TableView itself.
-
tableMenuButtonVisibleProperty
This controls whether a menu button is available when the user clicks in a designated space within the TableView, within which is a radio menu item for each TableColumn in this table. This menu allows for the user to show and hide all TableColumns easily. -
setTableMenuButtonVisible
public final void setTableMenuButtonVisible(boolean value)Sets the value of the property tableMenuButtonVisible.- Property description:
- This controls whether a menu button is available when the user clicks in a designated space within the TableView, within which is a radio menu item for each TableColumn in this table. This menu allows for the user to show and hide all TableColumns easily.
-
isTableMenuButtonVisible
public final boolean isTableMenuButtonVisible()Gets the value of the property tableMenuButtonVisible.- Property description:
- This controls whether a menu button is available when the user clicks in a designated space within the TableView, within which is a radio menu item for each TableColumn in this table. This menu allows for the user to show and hide all TableColumns easily.
-
setColumnResizePolicy
Sets the value of the property columnResizePolicy.- Property description:
- Called when the user completes a column-resize operation. The two most common
policies are available as static functions in the TableView class:
UNCONSTRAINED_RESIZE_POLICY
andCONSTRAINED_RESIZE_POLICY
.
-
getColumnResizePolicy
Gets the value of the property columnResizePolicy.- Property description:
- Called when the user completes a column-resize operation. The two most common
policies are available as static functions in the TableView class:
UNCONSTRAINED_RESIZE_POLICY
andCONSTRAINED_RESIZE_POLICY
.
-
columnResizePolicyProperty
public final ObjectProperty<Callback<TableView.ResizeFeatures,Boolean>> columnResizePolicyProperty()Called when the user completes a column-resize operation. The two most common policies are available as static functions in the TableView class:UNCONSTRAINED_RESIZE_POLICY
andCONSTRAINED_RESIZE_POLICY
. -
rowFactoryProperty
A function which produces a TableRow. The system is responsible for reusing TableRows. Return from this function a TableRow which might be usable for representing a single row in a TableView.Note that a TableRow is not a TableCell. A TableRow is simply a container for a TableCell, and in most circumstances it is more likely that you'll want to create custom TableCells, rather than TableRows. The primary use case for creating custom TableRow instances would most probably be to introduce some form of column spanning support.
You can create custom TableCell instances per column by assigning the appropriate function to the cellFactory property in the TableColumn class.
- See Also:
getRowFactory()
,setRowFactory(Callback)
-
setRowFactory
Sets the value of the property rowFactory.- Property description:
- A function which produces a TableRow. The system is responsible for
reusing TableRows. Return from this function a TableRow which
might be usable for representing a single row in a TableView.
Note that a TableRow is not a TableCell. A TableRow is simply a container for a TableCell, and in most circumstances it is more likely that you'll want to create custom TableCells, rather than TableRows. The primary use case for creating custom TableRow instances would most probably be to introduce some form of column spanning support.
You can create custom TableCell instances per column by assigning the appropriate function to the cellFactory property in the TableColumn class.
-
getRowFactory
Gets the value of the property rowFactory.- Property description:
- A function which produces a TableRow. The system is responsible for
reusing TableRows. Return from this function a TableRow which
might be usable for representing a single row in a TableView.
Note that a TableRow is not a TableCell. A TableRow is simply a container for a TableCell, and in most circumstances it is more likely that you'll want to create custom TableCells, rather than TableRows. The primary use case for creating custom TableRow instances would most probably be to introduce some form of column spanning support.
You can create custom TableCell instances per column by assigning the appropriate function to the cellFactory property in the TableColumn class.
-
placeholderProperty
This Node is shown to the user when the table has no content to show. This may be the case because the table model has no data in the first place, that a filter has been applied to the table model, resulting in there being nothing to show the user, or that there are no currently visible columns.- See Also:
getPlaceholder()
,setPlaceholder(Node)
-
setPlaceholder
Sets the value of the property placeholder.- Property description:
- This Node is shown to the user when the table has no content to show. This may be the case because the table model has no data in the first place, that a filter has been applied to the table model, resulting in there being nothing to show the user, or that there are no currently visible columns.
-
getPlaceholder
Gets the value of the property placeholder.- Property description:
- This Node is shown to the user when the table has no content to show. This may be the case because the table model has no data in the first place, that a filter has been applied to the table model, resulting in there being nothing to show the user, or that there are no currently visible columns.
-
selectionModelProperty
The SelectionModel provides the API through which it is possible to select single or multiple items within a TableView, as well as inspect which items have been selected by the user. Note that it has a generic type that must match the type of the TableView itself. -
setSelectionModel
Sets the value of the property selectionModel.- Property description:
- The SelectionModel provides the API through which it is possible to select single or multiple items within a TableView, as well as inspect which items have been selected by the user. Note that it has a generic type that must match the type of the TableView itself.
-
getSelectionModel
Gets the value of the property selectionModel.- Property description:
- The SelectionModel provides the API through which it is possible to select single or multiple items within a TableView, as well as inspect which items have been selected by the user. Note that it has a generic type that must match the type of the TableView itself.
-
setFocusModel
Sets the value of the property focusModel.- Property description:
- Represents the currently-installed
TableView.TableViewFocusModel
for this TableView. Under almost all circumstances leaving this as the default focus model will suffice.
-
getFocusModel
Gets the value of the property focusModel.- Property description:
- Represents the currently-installed
TableView.TableViewFocusModel
for this TableView. Under almost all circumstances leaving this as the default focus model will suffice.
-
focusModelProperty
Represents the currently-installedTableView.TableViewFocusModel
for this TableView. Under almost all circumstances leaving this as the default focus model will suffice. -
setEditable
public final void setEditable(boolean value)Sets the value of the property editable.- Property description:
- Specifies whether this TableView is editable - only if the TableView, the TableColumn (if applicable) and the TableCells within it are both editable will a TableCell be able to go into their editing state.
-
isEditable
public final boolean isEditable()Gets the value of the property editable.- Property description:
- Specifies whether this TableView is editable - only if the TableView, the TableColumn (if applicable) and the TableCells within it are both editable will a TableCell be able to go into their editing state.
-
editableProperty
Specifies whether this TableView is editable - only if the TableView, the TableColumn (if applicable) and the TableCells within it are both editable will a TableCell be able to go into their editing state.- See Also:
isEditable()
,setEditable(boolean)
-
setFixedCellSize
public final void setFixedCellSize(double value)Sets the new fixed cell size for this control. Any value greater than zero will enable fixed cell size mode, whereas a zero or negative value (or Region.USE_COMPUTED_SIZE) will be used to disabled fixed cell size mode.- Parameters:
value
- The new fixed cell size value, or a value less than or equal to zero (or Region.USE_COMPUTED_SIZE) to disable.- Since:
- JavaFX 8.0
-
getFixedCellSize
public final double getFixedCellSize()Returns the fixed cell size value. A value less than or equal to zero is used to represent that fixed cell size mode is disabled, and a value greater than zero represents the size of all cells in this control.- Returns:
- A double representing the fixed cell size of this control, or a value less than or equal to zero if fixed cell size mode is disabled.
- Since:
- JavaFX 8.0
-
fixedCellSizeProperty
Specifies whether this control has cells that are a fixed height (of the specified value). If this value is less than or equal to zero, then all cells are individually sized and positioned. This is a slow operation. Therefore, when performance matters and developers are not dependent on variable cell sizes it is a good idea to set the fixed cell size value. Generally cells are around 24px, so setting a fixed cell size of 24 is likely to result in very little difference in visuals, but a improvement to performance.To set this property via CSS, use the -fx-fixed-cell-size property. This should not be confused with the -fx-cell-size property. The difference between these two CSS properties is that -fx-cell-size will size all cells to the specified size, but it will not enforce that this is the only size (thus allowing for variable cell sizes, and preventing the performance gains from being possible). Therefore, when performance matters use -fx-fixed-cell-size, instead of -fx-cell-size. If both properties are specified in CSS, -fx-fixed-cell-size takes precedence.
- Since:
- JavaFX 8.0
- See Also:
getFixedCellSize()
,setFixedCellSize(double)
-
getEditingCell
Gets the value of the property editingCell.- Property description:
- Represents the current cell being edited, or null if there is no cell being edited.
-
editingCellProperty
Represents the current cell being edited, or null if there is no cell being edited.- See Also:
getEditingCell()
-
getComparator
Gets the value of the property comparator.- Property description:
- The comparator property is a read-only property that is representative of the
current state of the
sort order
list. The sort order list contains the columns that have been added to it either programmatically or via a user clicking on the headers themselves. - Since:
- JavaFX 8.0
-
comparatorProperty
The comparator property is a read-only property that is representative of the current state of thesort order
list. The sort order list contains the columns that have been added to it either programmatically or via a user clicking on the headers themselves.- Since:
- JavaFX 8.0
- See Also:
getComparator()
-
setSortPolicy
Sets the value of the property sortPolicy.- Property description:
- The sort policy specifies how sorting in this TableView should be performed.
For example, a basic sort policy may just call
FXCollections.sort(tableView.getItems())
, whereas a more advanced sort policy may call to a database to perform the necessary sorting on the server-side.TableView ships with a
default sort policy
that does precisely as mentioned above: it simply attempts to sort the items list in-place.It is recommended that rather than override the
sort
method that a different sort policy be provided instead. - Since:
- JavaFX 8.0
-
getSortPolicy
Gets the value of the property sortPolicy.- Property description:
- The sort policy specifies how sorting in this TableView should be performed.
For example, a basic sort policy may just call
FXCollections.sort(tableView.getItems())
, whereas a more advanced sort policy may call to a database to perform the necessary sorting on the server-side.TableView ships with a
default sort policy
that does precisely as mentioned above: it simply attempts to sort the items list in-place.It is recommended that rather than override the
sort
method that a different sort policy be provided instead. - Since:
- JavaFX 8.0
-
sortPolicyProperty
The sort policy specifies how sorting in this TableView should be performed. For example, a basic sort policy may just callFXCollections.sort(tableView.getItems())
, whereas a more advanced sort policy may call to a database to perform the necessary sorting on the server-side.TableView ships with a
default sort policy
that does precisely as mentioned above: it simply attempts to sort the items list in-place.It is recommended that rather than override the
sort
method that a different sort policy be provided instead.- Since:
- JavaFX 8.0
- See Also:
getSortPolicy()
,setSortPolicy(Callback)
-
setOnSort
Sets the value of the property onSort.- Property description:
- Called when there's a request to sort the control.
- Since:
- JavaFX 8.0
-
getOnSort
Gets the value of the property onSort.- Property description:
- Called when there's a request to sort the control.
- Since:
- JavaFX 8.0
-
onSortProperty
Called when there's a request to sort the control.- Since:
- JavaFX 8.0
- See Also:
getOnSort()
,setOnSort(EventHandler)
-
getColumns
The TableColumns that are part of this TableView. As the user reorders the TableView columns, this list will be updated to reflect the current visual ordering.Note: to display any data in a TableView, there must be at least one TableColumn in this ObservableList.
- Returns:
- the columns
-
getSortOrder
The sortOrder list defines the order in whichTableColumn
instances are sorted. An empty sortOrder list means that no sorting is being applied on the TableView. If the sortOrder list has one TableColumn within it, the TableView will be sorted using thesortType
andcomparator
properties of this TableColumn (assumingTableColumn.sortable
is true). If the sortOrder list contains multiple TableColumn instances, then the TableView is firstly sorted based on the properties of the first TableColumn. If two elements are considered equal, then the second TableColumn in the list is used to determine ordering. This repeats until the results from all TableColumn comparators are considered, if necessary.- Returns:
- An ObservableList containing zero or more TableColumn instances.
-
scrollTo
public void scrollTo(int index)Scrolls the TableView so that the given index is visible within the viewport.- Parameters:
index
- The index of an item that should be visible to the user.
-
scrollTo
Scrolls the TableView so that the given object is visible within the viewport.- Parameters:
object
- The object that should be visible to the user.- Since:
- JavaFX 8.0
-
setOnScrollTo
Sets the value of the property onScrollTo.- Property description:
- Called when there's a request to scroll an index into view using
scrollTo(int)
orscrollTo(Object)
- Since:
- JavaFX 8.0
-
getOnScrollTo
Gets the value of the property onScrollTo.- Property description:
- Called when there's a request to scroll an index into view using
scrollTo(int)
orscrollTo(Object)
- Since:
- JavaFX 8.0
-
onScrollToProperty
Called when there's a request to scroll an index into view usingscrollTo(int)
orscrollTo(Object)
- Since:
- JavaFX 8.0
- See Also:
getOnScrollTo()
,setOnScrollTo(EventHandler)
-
scrollToColumn
Scrolls the TableView so that the given column is visible within the viewport.- Parameters:
column
- The column that should be visible to the user.- Since:
- JavaFX 8.0
-
scrollToColumnIndex
public void scrollToColumnIndex(int columnIndex)Scrolls the TableView so that the given index is visible within the viewport.- Parameters:
columnIndex
- The index of a column that should be visible to the user.- Since:
- JavaFX 8.0
-
setOnScrollToColumn
Sets the value of the property onScrollToColumn.- Property description:
- Called when there's a request to scroll a column into view using
scrollToColumn(TableColumn)
orscrollToColumnIndex(int)
- Since:
- JavaFX 8.0
-
getOnScrollToColumn
Gets the value of the property onScrollToColumn.- Property description:
- Called when there's a request to scroll a column into view using
scrollToColumn(TableColumn)
orscrollToColumnIndex(int)
- Since:
- JavaFX 8.0
-
onScrollToColumnProperty
Called when there's a request to scroll a column into view usingscrollToColumn(TableColumn)
orscrollToColumnIndex(int)
- Since:
- JavaFX 8.0
- See Also:
getOnScrollToColumn()
,setOnScrollToColumn(EventHandler)
-
resizeColumn
Applies the currently installed resize policy against the given column, resizing it based on the delta value provided.- Parameters:
column
- the columndelta
- the delta- Returns:
- true if column resize is allowed
-
edit
Causes the cell at the given row/column view indexes to switch into its editing state, if it is not already in it, and assuming that the TableView and column are also editable.Note: This method will cancel editing if the given row value is less than zero and the given column is null.
- Parameters:
row
- the rowcolumn
- the column
-
getVisibleLeafColumns
Returns an unmodifiable list containing the currently visible leaf columns.- Returns:
- an unmodifiable list containing the currently visible leaf columns
-
getVisibleLeafIndex
Returns the position of the given column, relative to all other visible leaf columns.- Parameters:
column
- the column- Returns:
- the position of the given column, relative to all other visible leaf columns
-
getVisibleLeafColumn
Returns the TableColumn in the given column index, relative to all other visible leaf columns.- Parameters:
column
- the column- Returns:
- the TableColumn in the given column index, relative to all other visible leaf columns
-
createDefaultSkin
Create a new instance of the default skin for this control. This is called to create a skin for the control if no skin is provided via CSS-fx-skin
or set explicitly in a sub-class withsetSkin(...)
.- Overrides:
createDefaultSkin
in classControl
- Returns:
- new instance of default skin for this control. If null then the control will have no skin unless one is provided by css.
-
sort
public void sort()The sort method forces the TableView to re-run its sorting algorithm. More often than not it is not necessary to call this method directly, as it is automatically called when thesort order
,sort policy
, or the state of the TableColumnsort type
properties change. In other words, this method should only be called directly when something external changes and a sort is required.- Since:
- JavaFX 8.0
-
refresh
public void refresh()Callingrefresh()
forces the TableView control to recreate and repopulate the cells necessary to populate the visual bounds of the control. In other words, this forces the TableView to update what it is showing to the user. This is useful in cases where the underlying data source has changed in a way that is not observed by the TableView itself.- Since:
- JavaFX 8u60
-
getClassCssMetaData
- Returns:
- The CssMetaData associated with this class, which may include the CssMetaData of its superclasses.
- Since:
- JavaFX 8.0
-
getControlCssMetaData
- Overrides:
getControlCssMetaData
in classControl
- Returns:
- unmodifiable list of the controls css styleable properties
- Since:
- JavaFX 8.0
-
queryAccessibleAttribute
This method is called by the assistive technology to request the value for an attribute.This method is commonly overridden by subclasses to implement attributes that are required for a specific role.
If a particular attribute is not handled, the superclass implementation must be called.- Overrides:
queryAccessibleAttribute
in classControl
- Parameters:
attribute
- the requested attributeparameters
- optional list of parameters- Returns:
- the value for the requested attribute
- See Also:
AccessibleAttribute
-