- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
The three fundamental variables of the slider are min
,
max
, and value
. The value
should always
be a number within the range defined by min
and
max
. min
should always be less than or equal to
max
(although a slider whose min
and
max
are equal is a degenerate case that makes no sense).
min
defaults to 0, whereas max
defaults to 100.
This first example creates a slider whose range, or span, goes from 0 to 1, and whose value defaults to .5:
Slider slider = new Slider(0, 1, 0.5);
This next example shows a slider with customized tick marks and tick mark labels, which also spans from 0 to 1:
Slider slider = new Slider(0, 1, 0.5); slider.setShowTickMarks(true); slider.setShowTickLabels(true); slider.setMajorTickUnit(0.25f); slider.setBlockIncrement(0.1f);
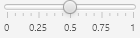
- Since:
- JavaFX 2.0
-
Property Summary
PropertiesTypePropertyDescriptionfinal DoubleProperty
The amount by which to adjust the slider if the track of the slider is clicked.final ObjectProperty<StringConverter<Double>>
A function for formatting the label for a major tick.final DoubleProperty
The unit distance between major tick marks.final DoubleProperty
The maximum value represented by this Slider.final IntegerProperty
The number of minor ticks to place between any two major ticks.final DoubleProperty
The minimum value represented by this Slider.final ObjectProperty<Orientation>
The orientation of theSlider
can either be horizontal or vertical.final BooleanProperty
Indicates that the labels for tick marks should be shown.final BooleanProperty
Specifies whether theSkin
implementation should show tick marks.final BooleanProperty
Indicates whether thevalue
of theSlider
should always be aligned with the tick marks.final BooleanProperty
When true, indicates the current value of this Slider is changing.final DoubleProperty
The current value represented by this Slider.Properties declared in class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties declared in class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties declared in class javafx.scene.Parent
needsLayout
Properties declared in class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, focusVisible, focusWithin, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Field Summary
Fields declared in class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields declared in class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionvoid
adjustValue
(double newValue) Adjustsvalue
to matchnewValue
.final DoubleProperty
The amount by which to adjust the slider if the track of the slider is clicked.void
Decrements the value byblockIncrement
, bounded by max.final double
Gets the value of theblockIncrement
property.static List<CssMetaData<? extends Styleable,
?>> Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.protected List<CssMetaData<? extends Styleable,
?>> Gets the unmodifiable list of the control's CSS-styleable properties.final StringConverter<Double>
Gets the value of thelabelFormatter
property.final double
Gets the value of themajorTickUnit
property.final double
getMax()
Gets the value of themax
property.final double
getMin()
Gets the value of themin
property.final int
Gets the value of theminorTickCount
property.final Orientation
Gets the value of theorientation
property.final double
getValue()
Gets the value of thevalue
property.void
Increments the value byblockIncrement
, bounded by max.final boolean
Gets the value of theshowTickLabels
property.final boolean
Gets the value of theshowTickMarks
property.final boolean
Gets the value of thesnapToTicks
property.final boolean
Gets the value of thevalueChanging
property.final ObjectProperty<StringConverter<Double>>
A function for formatting the label for a major tick.final DoubleProperty
The unit distance between major tick marks.final DoubleProperty
The maximum value represented by this Slider.final IntegerProperty
The number of minor ticks to place between any two major ticks.final DoubleProperty
The minimum value represented by this Slider.final ObjectProperty<Orientation>
The orientation of theSlider
can either be horizontal or vertical.final void
setBlockIncrement
(double value) Sets the value of theblockIncrement
property.final void
setLabelFormatter
(StringConverter<Double> value) Sets the value of thelabelFormatter
property.final void
setMajorTickUnit
(double value) Sets the value of themajorTickUnit
property.final void
setMax
(double value) Sets the value of themax
property.final void
setMin
(double value) Sets the value of themin
property.final void
setMinorTickCount
(int value) Sets the value of theminorTickCount
property.final void
setOrientation
(Orientation value) Sets the value of theorientation
property.final void
setShowTickLabels
(boolean value) Sets the value of theshowTickLabels
property.final void
setShowTickMarks
(boolean value) Sets the value of theshowTickMarks
property.final void
setSnapToTicks
(boolean value) Sets the value of thesnapToTicks
property.final void
setValue
(double value) Sets the value of thevalue
property.final void
setValueChanging
(boolean value) Sets the value of thevalueChanging
property.final BooleanProperty
Indicates that the labels for tick marks should be shown.final BooleanProperty
Specifies whether theSkin
implementation should show tick marks.final BooleanProperty
Indicates whether thevalue
of theSlider
should always be aligned with the tick marks.final BooleanProperty
When true, indicates the current value of this Slider is changing.final DoubleProperty
The current value represented by this Slider.Methods declared in class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, contextMenuProperty, createDefaultSkin, getContextMenu, getCssMetaData, getInitialFocusTraversable, getSkin, getTooltip, isResizable, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods declared in class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, computePrefHeight, computePrefWidth, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods declared in class javafx.scene.Parent
getBaselineOffset, getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, layoutChildren, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods declared in class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, focusVisibleProperty, focusWithinProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isFocusVisible, isFocusWithin, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
max
The maximum value represented by this Slider. This must be a value greater thanmin
.- See Also:
-
min
The minimum value represented by this Slider. This must be a value less thanmax
.- See Also:
-
value
The current value represented by this Slider. This value must always be betweenmin
andmax
, inclusive. If it is ever out of bounds either due tomin
ormax
changing or due to itself being changed, then it will be clamped to always remain valid.- See Also:
-
valueChanging
When true, indicates the current value of this Slider is changing. It provides notification that the value is changing. Once the value is computed, it is reset back to false. -
orientation
The orientation of theSlider
can either be horizontal or vertical. -
showTickLabels
Indicates that the labels for tick marks should be shown. Typically aSkin
implementation will only show labels ifshowTickMarks
is also true. -
showTickMarks
Specifies whether theSkin
implementation should show tick marks. -
majorTickUnit
The unit distance between major tick marks. For example, if themin
is 0 and themax
is 100 and themajorTickUnit
is 25, then there would be 5 tick marks: one at position 0, one at position 25, one at position 50, one at position 75, and a final one at position 100.This value should be positive and should be a value less than the span. Out of range values are essentially the same as disabling tick marks.
-
minorTickCount
The number of minor ticks to place between any two major ticks. This number should be positive or zero. Out of range values will disable disable minor ticks, as will a value of zero. -
snapToTicks
Indicates whether thevalue
of theSlider
should always be aligned with the tick marks. This is honored even if the tick marks are not shown. -
labelFormatter
A function for formatting the label for a major tick. The number representing the major tick will be passed to the function. If this function is not specified, then a default function will be used by theSkin
implementation. -
blockIncrement
The amount by which to adjust the slider if the track of the slider is clicked. This is used when manipulating the slider position using keys. IfsnapToTicks
is true then the nearest tick mark to the adjusted value will be used.
-
-
Constructor Details
-
Slider
public Slider()Creates a default Slider instance. -
Slider
public Slider(double min, double max, double value) Constructs a Slider control with the specified slider min, max and current value values.- Parameters:
min
- Slider minimum valuemax
- Slider maximum valuevalue
- Slider current value
-
-
Method Details
-
setMax
public final void setMax(double value) Sets the value of themax
property.- Property description:
- The maximum value represented by this Slider. This must be a
value greater than
min
. - Parameters:
value
- the value for themax
property- See Also:
-
getMax
public final double getMax()Gets the value of themax
property.- Property description:
- The maximum value represented by this Slider. This must be a
value greater than
min
. - Returns:
- the value of the
max
property - See Also:
-
maxProperty
The maximum value represented by this Slider. This must be a value greater thanmin
.- Returns:
- the
max
property - See Also:
-
setMin
public final void setMin(double value) Sets the value of themin
property.- Property description:
- The minimum value represented by this Slider. This must be a
value less than
max
. - Parameters:
value
- the value for themin
property- See Also:
-
getMin
public final double getMin()Gets the value of themin
property.- Property description:
- The minimum value represented by this Slider. This must be a
value less than
max
. - Returns:
- the value of the
min
property - See Also:
-
minProperty
The minimum value represented by this Slider. This must be a value less thanmax
.- Returns:
- the
min
property - See Also:
-
setValue
public final void setValue(double value) Sets the value of thevalue
property.- Property description:
- The current value represented by this Slider. This value must
always be between
min
andmax
, inclusive. If it is ever out of bounds either due tomin
ormax
changing or due to itself being changed, then it will be clamped to always remain valid. - Parameters:
value
- the value for thevalue
property- See Also:
-
getValue
public final double getValue()Gets the value of thevalue
property.- Property description:
- The current value represented by this Slider. This value must
always be between
min
andmax
, inclusive. If it is ever out of bounds either due tomin
ormax
changing or due to itself being changed, then it will be clamped to always remain valid. - Returns:
- the value of the
value
property - See Also:
-
valueProperty
The current value represented by this Slider. This value must always be betweenmin
andmax
, inclusive. If it is ever out of bounds either due tomin
ormax
changing or due to itself being changed, then it will be clamped to always remain valid.- Returns:
- the
value
property - See Also:
-
setValueChanging
public final void setValueChanging(boolean value) Sets the value of thevalueChanging
property.- Property description:
- When true, indicates the current value of this Slider is changing. It provides notification that the value is changing. Once the value is computed, it is reset back to false.
- Parameters:
value
- the value for thevalueChanging
property- See Also:
-
isValueChanging
public final boolean isValueChanging()Gets the value of thevalueChanging
property.- Property description:
- When true, indicates the current value of this Slider is changing. It provides notification that the value is changing. Once the value is computed, it is reset back to false.
- Returns:
- the value of the
valueChanging
property - See Also:
-
valueChangingProperty
When true, indicates the current value of this Slider is changing. It provides notification that the value is changing. Once the value is computed, it is reset back to false.- Returns:
- the
valueChanging
property - See Also:
-
setOrientation
Sets the value of theorientation
property.- Property description:
- The orientation of the
Slider
can either be horizontal or vertical. - Parameters:
value
- the value for theorientation
property- See Also:
-
getOrientation
Gets the value of theorientation
property.- Property description:
- The orientation of the
Slider
can either be horizontal or vertical. - Returns:
- the value of the
orientation
property - See Also:
-
orientationProperty
The orientation of theSlider
can either be horizontal or vertical.- Returns:
- the
orientation
property - See Also:
-
setShowTickLabels
public final void setShowTickLabels(boolean value) Sets the value of theshowTickLabels
property.- Property description:
- Indicates that the labels for tick marks should be shown. Typically a
Skin
implementation will only show labels ifshowTickMarks
is also true. - Parameters:
value
- the value for theshowTickLabels
property- See Also:
-
isShowTickLabels
public final boolean isShowTickLabels()Gets the value of theshowTickLabels
property.- Property description:
- Indicates that the labels for tick marks should be shown. Typically a
Skin
implementation will only show labels ifshowTickMarks
is also true. - Returns:
- the value of the
showTickLabels
property - See Also:
-
showTickLabelsProperty
Indicates that the labels for tick marks should be shown. Typically aSkin
implementation will only show labels ifshowTickMarks
is also true.- Returns:
- the
showTickLabels
property - See Also:
-
setShowTickMarks
public final void setShowTickMarks(boolean value) Sets the value of theshowTickMarks
property.- Property description:
- Specifies whether the
Skin
implementation should show tick marks. - Parameters:
value
- the value for theshowTickMarks
property- See Also:
-
isShowTickMarks
public final boolean isShowTickMarks()Gets the value of theshowTickMarks
property.- Property description:
- Specifies whether the
Skin
implementation should show tick marks. - Returns:
- the value of the
showTickMarks
property - See Also:
-
showTickMarksProperty
Specifies whether theSkin
implementation should show tick marks.- Returns:
- the
showTickMarks
property - See Also:
-
setMajorTickUnit
public final void setMajorTickUnit(double value) Sets the value of themajorTickUnit
property.- Property description:
- The unit distance between major tick marks. For example, if
the
min
is 0 and themax
is 100 and themajorTickUnit
is 25, then there would be 5 tick marks: one at position 0, one at position 25, one at position 50, one at position 75, and a final one at position 100.This value should be positive and should be a value less than the span. Out of range values are essentially the same as disabling tick marks.
- Parameters:
value
- the value for themajorTickUnit
property- See Also:
-
getMajorTickUnit
public final double getMajorTickUnit()Gets the value of themajorTickUnit
property.- Property description:
- The unit distance between major tick marks. For example, if
the
min
is 0 and themax
is 100 and themajorTickUnit
is 25, then there would be 5 tick marks: one at position 0, one at position 25, one at position 50, one at position 75, and a final one at position 100.This value should be positive and should be a value less than the span. Out of range values are essentially the same as disabling tick marks.
- Returns:
- the value of the
majorTickUnit
property - See Also:
-
majorTickUnitProperty
The unit distance between major tick marks. For example, if themin
is 0 and themax
is 100 and themajorTickUnit
is 25, then there would be 5 tick marks: one at position 0, one at position 25, one at position 50, one at position 75, and a final one at position 100.This value should be positive and should be a value less than the span. Out of range values are essentially the same as disabling tick marks.
- Returns:
- the
majorTickUnit
property - See Also:
-
setMinorTickCount
public final void setMinorTickCount(int value) Sets the value of theminorTickCount
property.- Property description:
- The number of minor ticks to place between any two major ticks. This number should be positive or zero. Out of range values will disable disable minor ticks, as will a value of zero.
- Parameters:
value
- the value for theminorTickCount
property- See Also:
-
getMinorTickCount
public final int getMinorTickCount()Gets the value of theminorTickCount
property.- Property description:
- The number of minor ticks to place between any two major ticks. This number should be positive or zero. Out of range values will disable disable minor ticks, as will a value of zero.
- Returns:
- the value of the
minorTickCount
property - See Also:
-
minorTickCountProperty
The number of minor ticks to place between any two major ticks. This number should be positive or zero. Out of range values will disable disable minor ticks, as will a value of zero.- Returns:
- the
minorTickCount
property - See Also:
-
setSnapToTicks
public final void setSnapToTicks(boolean value) Sets the value of thesnapToTicks
property.- Property description:
- Indicates whether the
value
of theSlider
should always be aligned with the tick marks. This is honored even if the tick marks are not shown. - Parameters:
value
- the value for thesnapToTicks
property- See Also:
-
isSnapToTicks
public final boolean isSnapToTicks()Gets the value of thesnapToTicks
property.- Property description:
- Indicates whether the
value
of theSlider
should always be aligned with the tick marks. This is honored even if the tick marks are not shown. - Returns:
- the value of the
snapToTicks
property - See Also:
-
snapToTicksProperty
Indicates whether thevalue
of theSlider
should always be aligned with the tick marks. This is honored even if the tick marks are not shown.- Returns:
- the
snapToTicks
property - See Also:
-
setLabelFormatter
Sets the value of thelabelFormatter
property.- Property description:
- A function for formatting the label for a major tick. The number
representing the major tick will be passed to the function. If this
function is not specified, then a default function will be used by
the
Skin
implementation. - Parameters:
value
- the value for thelabelFormatter
property- See Also:
-
getLabelFormatter
Gets the value of thelabelFormatter
property.- Property description:
- A function for formatting the label for a major tick. The number
representing the major tick will be passed to the function. If this
function is not specified, then a default function will be used by
the
Skin
implementation. - Returns:
- the value of the
labelFormatter
property - See Also:
-
labelFormatterProperty
A function for formatting the label for a major tick. The number representing the major tick will be passed to the function. If this function is not specified, then a default function will be used by theSkin
implementation.- Returns:
- the
labelFormatter
property - See Also:
-
setBlockIncrement
public final void setBlockIncrement(double value) Sets the value of theblockIncrement
property.- Property description:
- The amount by which to adjust the slider if the track of the slider is
clicked. This is used when manipulating the slider position using keys. If
snapToTicks
is true then the nearest tick mark to the adjusted value will be used. - Parameters:
value
- the value for theblockIncrement
property- See Also:
-
getBlockIncrement
public final double getBlockIncrement()Gets the value of theblockIncrement
property.- Property description:
- The amount by which to adjust the slider if the track of the slider is
clicked. This is used when manipulating the slider position using keys. If
snapToTicks
is true then the nearest tick mark to the adjusted value will be used. - Returns:
- the value of the
blockIncrement
property - See Also:
-
blockIncrementProperty
The amount by which to adjust the slider if the track of the slider is clicked. This is used when manipulating the slider position using keys. IfsnapToTicks
is true then the nearest tick mark to the adjusted value will be used.- Returns:
- the
blockIncrement
property - See Also:
-
adjustValue
public void adjustValue(double newValue) Adjustsvalue
to matchnewValue
. Thevalue
is the actual amount between themin
andmax
. This function also takes into accountsnapToTicks
, which is the main difference between adjustValue and setValue. It also ensures that the value is some valid number between min and max. Note: This function is intended to be used by experts, primarily by those implementing new Skins or Behaviors. It is not common for developers or designers to access this function directly.- Parameters:
newValue
- the new adjusted value
-
increment
public void increment()Increments the value byblockIncrement
, bounded by max. If the max is less than or equal to the min, then this method does nothing. -
decrement
public void decrement()Decrements the value byblockIncrement
, bounded by max. If the max is less than or equal to the min, then this method does nothing. -
getClassCssMetaData
Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.- Returns:
- the
CssMetaData
- Since:
- JavaFX 8.0
-
getControlCssMetaData
Gets the unmodifiable list of the control's CSS-styleable properties.- Overrides:
getControlCssMetaData
in classControl
- Returns:
- the unmodifiable list of the control's CSS-styleable properties
- Since:
- JavaFX 8.0
-