java.lang.Object
javafx.scene.Node
javafx.scene.Parent
javafx.scene.layout.Region
javafx.scene.control.Control
javafx.scene.control.Separator
- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
A horizontal or vertical separator line. The visual appearance of this
separator can be controlled via CSS. A horizontal separator occupies the
full horizontal space allocated to it (less padding), and a vertical
separator occupies the full vertical space allocated to it (less padding).
The 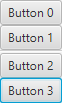
halignment
and valignment
properties determine how the
separator is positioned in the other dimension, for example, how a horizontal
separator is positioned vertically within its allocated space.
The separator is horizontal (i.e. isVertical() == false
) by default.
The style-class for this control is "separator".
The separator provides two pseudo-classes "horizontal" and "vertical" which are mutually exclusive. The "horizontal" pseudo-class applies if the separator is horizontal, and the "vertical" pseudo-class applies if the separator is vertical.
Separator sets focusTraversable to false.
Example:
Button b0 = new Button("Button 0");
Button b1 = new Button("Button 1");
Button b2 = new Button("Button 2");
Button b3 = new Button("Button 3");
Separator separator = new Separator(Orientation.HORIZONTAL);
VBox vBox = new VBox(b0, b1, separator, b2, b3);
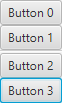
- Since:
- JavaFX 2.0
-
Property Summary
PropertiesTypePropertyDescriptionfinal ObjectProperty<HPos>
For vertical separators, specifies the horizontal position of the separator line within the separator control's space.final ObjectProperty<Orientation>
The orientation of theSeparator
can either be horizontal or vertical.final ObjectProperty<VPos>
For horizontal separators, specifies the vertical alignment of the separator line within the separator control's space.Properties declared in class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties declared in class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties declared in class javafx.scene.Parent
needsLayout
Properties declared in class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, focusVisible, focusWithin, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Field Summary
Fields declared in class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields declared in class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
ConstructorsConstructorDescriptionCreates a new horizontal separator with halignment and valignment set to their respective CENTER values.Separator
(Orientation orientation) Creates a new separator with halignment and valignment set to their respective CENTER values. -
Method Summary
Modifier and TypeMethodDescriptionstatic List<CssMetaData<? extends Styleable,
?>> Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.protected List<CssMetaData<? extends Styleable,
?>> Gets the unmodifiable list of the control's CSS-styleable properties.final HPos
Gets the value of thehalignment
property.protected Boolean
Returns the initial focus traversable state of this control, for use by the JavaFX CSS engine to correctly set its initial value.final Orientation
Gets the value of theorientation
property.final VPos
Gets the value of thevalignment
property.final ObjectProperty<HPos>
For vertical separators, specifies the horizontal position of the separator line within the separator control's space.final ObjectProperty<Orientation>
The orientation of theSeparator
can either be horizontal or vertical.final void
setHalignment
(HPos value) Sets the value of thehalignment
property.final void
setOrientation
(Orientation value) Sets the value of theorientation
property.final void
setValignment
(VPos value) Sets the value of thevalignment
property.final ObjectProperty<VPos>
For horizontal separators, specifies the vertical alignment of the separator line within the separator control's space.Methods declared in class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, contextMenuProperty, createDefaultSkin, getContextMenu, getCssMetaData, getSkin, getTooltip, isResizable, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods declared in class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, computePrefHeight, computePrefWidth, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods declared in class javafx.scene.Parent
getBaselineOffset, getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, layoutChildren, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods declared in class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, focusVisibleProperty, focusWithinProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isFocusVisible, isFocusWithin, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
orientation
The orientation of theSeparator
can either be horizontal or vertical. -
halignment
For vertical separators, specifies the horizontal position of the separator line within the separator control's space. Ignored for horizontal separators. -
valignment
For horizontal separators, specifies the vertical alignment of the separator line within the separator control's space. Ignored for vertical separators.
-
-
Constructor Details
-
Separator
public Separator()Creates a new horizontal separator with halignment and valignment set to their respective CENTER values. -
Separator
Creates a new separator with halignment and valignment set to their respective CENTER values. The direction of the separator is specified by the vertical property.- Parameters:
orientation
- Specifies whether the Separator instance is initially vertical or horizontal.
-
-
Method Details
-
setOrientation
Sets the value of theorientation
property.- Property description:
- The orientation of the
Separator
can either be horizontal or vertical. - Parameters:
value
- the value for theorientation
property- See Also:
-
getOrientation
Gets the value of theorientation
property.- Property description:
- The orientation of the
Separator
can either be horizontal or vertical. - Returns:
- the value of the
orientation
property - See Also:
-
orientationProperty
The orientation of theSeparator
can either be horizontal or vertical.- Returns:
- the
orientation
property - See Also:
-
setHalignment
Sets the value of thehalignment
property.- Property description:
- For vertical separators, specifies the horizontal position of the separator line within the separator control's space. Ignored for horizontal separators.
- Parameters:
value
- the value for thehalignment
property- See Also:
-
getHalignment
Gets the value of thehalignment
property.- Property description:
- For vertical separators, specifies the horizontal position of the separator line within the separator control's space. Ignored for horizontal separators.
- Returns:
- the value of the
halignment
property - See Also:
-
halignmentProperty
For vertical separators, specifies the horizontal position of the separator line within the separator control's space. Ignored for horizontal separators.- Returns:
- the
halignment
property - See Also:
-
setValignment
Sets the value of thevalignment
property.- Property description:
- For horizontal separators, specifies the vertical alignment of the separator line within the separator control's space. Ignored for vertical separators.
- Parameters:
value
- the value for thevalignment
property- See Also:
-
getValignment
Gets the value of thevalignment
property.- Property description:
- For horizontal separators, specifies the vertical alignment of the separator line within the separator control's space. Ignored for vertical separators.
- Returns:
- the value of the
valignment
property - See Also:
-
valignmentProperty
For horizontal separators, specifies the vertical alignment of the separator line within the separator control's space. Ignored for vertical separators.- Returns:
- the
valignment
property - See Also:
-
getClassCssMetaData
Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.- Returns:
- the
CssMetaData
- Since:
- JavaFX 8.0
-
getControlCssMetaData
Gets the unmodifiable list of the control's CSS-styleable properties.- Overrides:
getControlCssMetaData
in classControl
- Returns:
- the unmodifiable list of the control's CSS-styleable properties
- Since:
- JavaFX 8.0
-
getInitialFocusTraversable
Returns the initial focus traversable state of this control, for use by the JavaFX CSS engine to correctly set its initial value. This method is overridden as by default UI controls have focus traversable set to true, but that is not appropriate for this control.- Overrides:
getInitialFocusTraversable
in classControl
- Returns:
- the initial focus traversable state of this control
- Since:
- 9
-