- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
By default, the ChoiceBox has no item selected unless otherwise specified. Although the ChoiceBox will only allow a user to select from the predefined list, it is possible for the developer to specify the selected item to be something other than what is available in the predefined list. This is required for several important use cases. Configuration of the ChoiceBox is order independent. You may either specify the items and then the selected item, or you may specify the selected item and then the items. Either way will function correctly.
ChoiceBox item selection is handled by
SelectionModel
.
As with ListView and ComboBox, it is possible to modify the
SelectionModel
that is used,
although this is likely to be rarely changed. ChoiceBox supports only a
single selection model, hence the default used is a SingleSelectionModel
.
Example:
ChoiceBox cb = new ChoiceBox(); cb.getItems().addAll("item1", "item2", "item3");
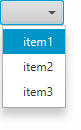
- Since:
- JavaFX 2.0
-
Property Summary
PropertiesTypePropertyDescriptionAllows a way to specify how to represent objects in the items list.final ObjectProperty<ObservableList<T>>
The items to display in the choice box.final ObjectProperty<EventHandler<ActionEvent>>
The ChoiceBox action, which is invoked whenever the ChoiceBoxvalue
property is changed.final ObjectProperty<EventHandler<Event>>
Called just after theChoiceBox
popup has been hidden.final ObjectProperty<EventHandler<Event>>
Called just prior to theChoiceBox
popup being hidden.final ObjectProperty<EventHandler<Event>>
Called just prior to theChoiceBox
popup being shown.final ObjectProperty<EventHandler<Event>>
Called just after theChoiceBox
popup is shown.final ObjectProperty<SingleSelectionModel<T>>
The selection model for the ChoiceBox.final ReadOnlyBooleanProperty
Indicates whether the drop down is displaying the list of choices to the user.The value of this ChoiceBox is defined as the selected item in the ChoiceBox selection model.Properties declared in class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties declared in class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties declared in class javafx.scene.Parent
needsLayout
Properties declared in class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Field Summary
FieldsModifier and TypeFieldDescriptionCalled when the ChoiceBox popup has been hidden.Called when the ChoiceBox popup will be hidden.Called prior to the ChoiceBox showing its popup after the user has clicked or otherwise interacted with the ChoiceBox.Called after the ChoiceBox has shown its popup.Fields declared in class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields declared in class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
ConstructorsConstructorDescriptionCreate a new ChoiceBox which has an empty list of items.ChoiceBox
(ObservableList<T> items) Create a new ChoiceBox with the given set of items. -
Method Summary
Modifier and TypeMethodDescriptionAllows a way to specify how to represent objects in the items list.final StringConverter<T>
Gets the value of the property converter.final ObservableList<T>
getItems()
Gets the value of the property items.final EventHandler<ActionEvent>
Gets the value of the property onAction.final EventHandler<Event>
Gets the value of the property onHidden.final EventHandler<Event>
Gets the value of the property onHiding.final EventHandler<Event>
Gets the value of the property onShowing.final EventHandler<Event>
Gets the value of the property onShown.final SingleSelectionModel<T>
Gets the value of the property selectionModel.final T
getValue()
Gets the value of the property value.void
hide()
Closes the list of choices.final boolean
Gets the value of the property showing.final ObjectProperty<ObservableList<T>>
The items to display in the choice box.final ObjectProperty<EventHandler<ActionEvent>>
The ChoiceBox action, which is invoked whenever the ChoiceBoxvalue
property is changed.final ObjectProperty<EventHandler<Event>>
Called just after theChoiceBox
popup has been hidden.final ObjectProperty<EventHandler<Event>>
Called just prior to theChoiceBox
popup being hidden.final ObjectProperty<EventHandler<Event>>
Called just prior to theChoiceBox
popup being shown.final ObjectProperty<EventHandler<Event>>
Called just after theChoiceBox
popup is shown.final ObjectProperty<SingleSelectionModel<T>>
The selection model for the ChoiceBox.final void
setConverter
(StringConverter<T> value) Sets the value of the property converter.final void
setItems
(ObservableList<T> value) Sets the value of the property items.final void
setOnAction
(EventHandler<ActionEvent> value) Sets the value of the property onAction.final void
setOnHidden
(EventHandler<Event> value) Sets the value of the property onHidden.final void
setOnHiding
(EventHandler<Event> value) Sets the value of the property onHiding.final void
setOnShowing
(EventHandler<Event> value) Sets the value of the property onShowing.final void
setOnShown
(EventHandler<Event> value) Sets the value of the property onShown.final void
setSelectionModel
(SingleSelectionModel<T> value) Sets the value of the property selectionModel.final void
Sets the value of the property value.void
show()
Opens the list of choices.final ReadOnlyBooleanProperty
Indicates whether the drop down is displaying the list of choices to the user.The value of this ChoiceBox is defined as the selected item in the ChoiceBox selection model.Methods declared in class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, contextMenuProperty, createDefaultSkin, getClassCssMetaData, getContextMenu, getControlCssMetaData, getCssMetaData, getInitialFocusTraversable, getSkin, getTooltip, isResizable, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods declared in class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, computePrefHeight, computePrefWidth, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods declared in class javafx.scene.Parent
getBaselineOffset, getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, layoutChildren, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods declared in class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
selectionModel
The selection model for the ChoiceBox. Only a single choice can be made, hence, the ChoiceBox supports only a SingleSelectionModel. Generally, the main interaction with the selection model is to explicitly set which item in the items list should be selected, or to listen to changes in the selection to know which item has been chosen. -
showing
Indicates whether the drop down is displaying the list of choices to the user. This is a readonly property which should be manipulated by means of the #show and #hide methods.- See Also:
-
items
The items to display in the choice box. The selected item (as indicated in the selection model) must always be one of these items.- See Also:
-
converter
Allows a way to specify how to represent objects in the items list. When a StringConverter is set, the object toString method is not called and instead its toString(object T) is called, passing the objects in the items list. This is useful when using domain objects in a ChoiceBox as this property allows for customization of the representation. Also, any of the pre-built Converters available in thejavafx.util.converter
package can be set.- Since:
- JavaFX 2.1
- See Also:
-
value
The value of this ChoiceBox is defined as the selected item in the ChoiceBox selection model. The valueProperty is synchronized with the selectedItem. This property allows for bi-directional binding of external properties to the ChoiceBox and updates the selection model accordingly.- Since:
- JavaFX 2.1
- See Also:
-
onAction
The ChoiceBox action, which is invoked whenever the ChoiceBoxvalue
property is changed. This may be due to the value property being programmatically changed or when the user selects an item in a popup menu.- Since:
- JavaFX 8u60
- See Also:
-
onShowing
Called just prior to theChoiceBox
popup being shown.- Since:
- JavaFX 8u60
- See Also:
-
onShown
Called just after theChoiceBox
popup is shown.- Since:
- JavaFX 8u60
- See Also:
-
onHiding
Called just prior to theChoiceBox
popup being hidden.- Since:
- JavaFX 8u60
- See Also:
-
onHidden
Called just after theChoiceBox
popup has been hidden.- Since:
- JavaFX 8u60
- See Also:
-
-
Field Details
-
ON_SHOWING
Called prior to the ChoiceBox showing its popup after the user has clicked or otherwise interacted with the ChoiceBox.- Since:
- JavaFX 8u60
-
ON_SHOWN
Called after the ChoiceBox has shown its popup.- Since:
- JavaFX 8u60
-
ON_HIDING
Called when the ChoiceBox popup will be hidden.- Since:
- JavaFX 8u60
-
ON_HIDDEN
Called when the ChoiceBox popup has been hidden.- Since:
- JavaFX 8u60
-
-
Constructor Details
-
ChoiceBox
public ChoiceBox()Create a new ChoiceBox which has an empty list of items. -
ChoiceBox
Create a new ChoiceBox with the given set of items. Since it is observable, the content of this list may change over time and the ChoiceBox will be updated accordingly.- Parameters:
items
- the set of items
-
-
Method Details
-
setSelectionModel
Sets the value of the property selectionModel.- Property description:
- The selection model for the ChoiceBox. Only a single choice can be made, hence, the ChoiceBox supports only a SingleSelectionModel. Generally, the main interaction with the selection model is to explicitly set which item in the items list should be selected, or to listen to changes in the selection to know which item has been chosen.
-
getSelectionModel
Gets the value of the property selectionModel.- Property description:
- The selection model for the ChoiceBox. Only a single choice can be made, hence, the ChoiceBox supports only a SingleSelectionModel. Generally, the main interaction with the selection model is to explicitly set which item in the items list should be selected, or to listen to changes in the selection to know which item has been chosen.
-
selectionModelProperty
The selection model for the ChoiceBox. Only a single choice can be made, hence, the ChoiceBox supports only a SingleSelectionModel. Generally, the main interaction with the selection model is to explicitly set which item in the items list should be selected, or to listen to changes in the selection to know which item has been chosen. -
isShowing
public final boolean isShowing()Gets the value of the property showing.- Property description:
- Indicates whether the drop down is displaying the list of choices to the user. This is a readonly property which should be manipulated by means of the #show and #hide methods.
-
showingProperty
Indicates whether the drop down is displaying the list of choices to the user. This is a readonly property which should be manipulated by means of the #show and #hide methods.- See Also:
-
setItems
Sets the value of the property items.- Property description:
- The items to display in the choice box. The selected item (as indicated in the selection model) must always be one of these items.
-
getItems
Gets the value of the property items.- Property description:
- The items to display in the choice box. The selected item (as indicated in the selection model) must always be one of these items.
-
itemsProperty
The items to display in the choice box. The selected item (as indicated in the selection model) must always be one of these items.- See Also:
-
converterProperty
Allows a way to specify how to represent objects in the items list. When a StringConverter is set, the object toString method is not called and instead its toString(object T) is called, passing the objects in the items list. This is useful when using domain objects in a ChoiceBox as this property allows for customization of the representation. Also, any of the pre-built Converters available in thejavafx.util.converter
package can be set.- Since:
- JavaFX 2.1
- See Also:
-
setConverter
Sets the value of the property converter.- Property description:
- Allows a way to specify how to represent objects in the items list. When
a StringConverter is set, the object toString method is not called and
instead its toString(object T) is called, passing the objects in the items list.
This is useful when using domain objects in a ChoiceBox as this property
allows for customization of the representation. Also, any of the pre-built
Converters available in the
javafx.util.converter
package can be set. - Since:
- JavaFX 2.1
-
getConverter
Gets the value of the property converter.- Property description:
- Allows a way to specify how to represent objects in the items list. When
a StringConverter is set, the object toString method is not called and
instead its toString(object T) is called, passing the objects in the items list.
This is useful when using domain objects in a ChoiceBox as this property
allows for customization of the representation. Also, any of the pre-built
Converters available in the
javafx.util.converter
package can be set. - Since:
- JavaFX 2.1
-
valueProperty
The value of this ChoiceBox is defined as the selected item in the ChoiceBox selection model. The valueProperty is synchronized with the selectedItem. This property allows for bi-directional binding of external properties to the ChoiceBox and updates the selection model accordingly.- Since:
- JavaFX 2.1
- See Also:
-
setValue
Sets the value of the property value.- Property description:
- The value of this ChoiceBox is defined as the selected item in the ChoiceBox selection model. The valueProperty is synchronized with the selectedItem. This property allows for bi-directional binding of external properties to the ChoiceBox and updates the selection model accordingly.
- Since:
- JavaFX 2.1
-
getValue
Gets the value of the property value.- Property description:
- The value of this ChoiceBox is defined as the selected item in the ChoiceBox selection model. The valueProperty is synchronized with the selectedItem. This property allows for bi-directional binding of external properties to the ChoiceBox and updates the selection model accordingly.
- Since:
- JavaFX 2.1
-
onActionProperty
The ChoiceBox action, which is invoked whenever the ChoiceBoxvalue
property is changed. This may be due to the value property being programmatically changed or when the user selects an item in a popup menu.- Since:
- JavaFX 8u60
- See Also:
-
setOnAction
Sets the value of the property onAction.- Property description:
- The ChoiceBox action, which is invoked whenever the ChoiceBox
value
property is changed. This may be due to the value property being programmatically changed or when the user selects an item in a popup menu. - Since:
- JavaFX 8u60
-
getOnAction
Gets the value of the property onAction.- Property description:
- The ChoiceBox action, which is invoked whenever the ChoiceBox
value
property is changed. This may be due to the value property being programmatically changed or when the user selects an item in a popup menu. - Since:
- JavaFX 8u60
-
onShowingProperty
Called just prior to theChoiceBox
popup being shown.- Since:
- JavaFX 8u60
- See Also:
-
setOnShowing
Sets the value of the property onShowing.- Property description:
- Called just prior to the
ChoiceBox
popup being shown. - Since:
- JavaFX 8u60
-
getOnShowing
Gets the value of the property onShowing.- Property description:
- Called just prior to the
ChoiceBox
popup being shown. - Since:
- JavaFX 8u60
-
onShownProperty
Called just after theChoiceBox
popup is shown.- Since:
- JavaFX 8u60
- See Also:
-
setOnShown
Sets the value of the property onShown.- Property description:
- Called just after the
ChoiceBox
popup is shown. - Since:
- JavaFX 8u60
-
getOnShown
Gets the value of the property onShown.- Property description:
- Called just after the
ChoiceBox
popup is shown. - Since:
- JavaFX 8u60
-
onHidingProperty
Called just prior to theChoiceBox
popup being hidden.- Since:
- JavaFX 8u60
- See Also:
-
setOnHiding
Sets the value of the property onHiding.- Property description:
- Called just prior to the
ChoiceBox
popup being hidden. - Since:
- JavaFX 8u60
-
getOnHiding
Gets the value of the property onHiding.- Property description:
- Called just prior to the
ChoiceBox
popup being hidden. - Since:
- JavaFX 8u60
-
onHiddenProperty
Called just after theChoiceBox
popup has been hidden.- Since:
- JavaFX 8u60
- See Also:
-
setOnHidden
Sets the value of the property onHidden.- Property description:
- Called just after the
ChoiceBox
popup has been hidden. - Since:
- JavaFX 8u60
-
getOnHidden
Gets the value of the property onHidden.- Property description:
- Called just after the
ChoiceBox
popup has been hidden. - Since:
- JavaFX 8u60
-
show
public void show()Opens the list of choices. -
hide
public void hide()Closes the list of choices.
-