- All Implemented Interfaces:
EventTarget
Dialog
class, and provides support for a number
of pre-built dialog types that can be easily shown to users to prompt for a
response. Therefore, for many users, the Alert class is the most suited class
for their needs (as opposed to using Dialog
directly). Alternatively,
users who want to prompt a user for text input or to make a choice from a list
of options would be better served by using TextInputDialog
and
ChoiceDialog
, respectively.
When creating an Alert instance, users must pass in an Alert.AlertType
enumeration value. It is by passing in this value that the Alert instance will
configure itself appropriately (by setting default values for many of the
Dialog
properties, including title
,
header
, and graphic
,
as well as the default buttons
that are expected in
a dialog of the given type.
To instantiate (but not yet show) an Alert, simply use code such as the following:
Alert alert = new Alert(AlertType.CONFIRMATION, "Are you sure you want to format your system?");
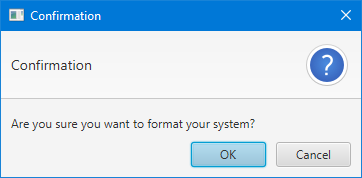
Once an Alert is instantiated, we must show it. More often than not, alerts (and dialogs in general) are shown in a modal and blocking fashion. 'Modal' means that the dialog prevents user interaction with the owning application whilst it is showing, and 'blocking' means that code execution stops at the point in which the dialog is shown. This means that you can show a dialog, await the user response, and then continue running the code that directly follows the show call, giving developers the ability to immediately deal with the user input from the dialog (if relevant).
JavaFX dialogs are modal by default (you can change this via the
Dialog.initModality(javafx.stage.Modality)
API). To specify whether you want
blocking or non-blocking dialogs, developers simply choose to call
Dialog.showAndWait()
or Dialog.show()
(respectively). By default most
developers should choose to use Dialog.showAndWait()
, given the ease of
coding in these situations. Shown below is three code snippets, showing three
equally valid ways of showing the Alert dialog that was specified above:
Option 1: The 'traditional' approach
Optional<ButtonType> result = alert.showAndWait();
if (result.isPresent() && result.get() == ButtonType.OK) {
formatSystem();
}
Option 2: The traditional + Optional approach
alert.showAndWait().ifPresent(response -> {
if (response == ButtonType.OK) {
formatSystem();
}
});
Option 3: The fully lambda approach
alert.showAndWait()
.filter(response -> response == ButtonType.OK)
.ifPresent(response -> formatSystem());
There is no better or worse option of the three listed above, so developers
are encouraged to work to their own style preferences. The purpose of showing
the above is to help introduce developers to the Optional
API, which
is new in Java 8 and may be foreign to many developers.
- Since:
- JavaFX 8u40
- See Also:
-
Property Summary
PropertiesTypePropertyDescriptionfinal ObjectProperty<Alert.AlertType>
When creating an Alert instance, users must pass in anAlert.AlertType
enumeration value.Properties declared in class javafx.scene.control.Dialog
contentText, dialogPane, graphic, headerText, height, onCloseRequest, onHidden, onHiding, onShowing, onShown, resizable, resultConverter, result, showing, title, width, x, y
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
An enumeration containing the available, pre-built alert types that theAlert
class can use to pre-populate various properties. -
Constructor Summary
ConstructorsConstructorDescriptionAlert
(Alert.AlertType alertType) Creates an alert with the given AlertType (refer to theAlert.AlertType
documentation for clarification over which one is most appropriate).Alert
(Alert.AlertType alertType, String contentText, ButtonType... buttons) Creates an alert with the given contentText, ButtonTypes, and AlertType (refer to theAlert.AlertType
documentation for clarification over which one is most appropriate). -
Method Summary
Modifier and TypeMethodDescriptionfinal ObjectProperty<Alert.AlertType>
When creating an Alert instance, users must pass in anAlert.AlertType
enumeration value.final Alert.AlertType
Gets the value of the property alertType.final ObservableList<ButtonType>
Returns anObservableList
of allButtonType
instances that are currently set inside this Alert instance.final void
setAlertType
(Alert.AlertType alertType) Sets the value of the property alertType.Methods declared in class javafx.scene.control.Dialog
buildEventDispatchChain, close, contentTextProperty, dialogPaneProperty, getContentText, getDialogPane, getGraphic, getHeaderText, getHeight, getModality, getOnCloseRequest, getOnHidden, getOnHiding, getOnShowing, getOnShown, getOwner, getResult, getResultConverter, getTitle, getWidth, getX, getY, graphicProperty, headerTextProperty, heightProperty, hide, initModality, initOwner, initStyle, isResizable, isShowing, onCloseRequestProperty, onHiddenProperty, onHidingProperty, onShowingProperty, onShownProperty, resizableProperty, resultConverterProperty, resultProperty, setContentText, setDialogPane, setGraphic, setHeaderText, setHeight, setOnCloseRequest, setOnHidden, setOnHiding, setOnShowing, setOnShown, setResizable, setResult, setResultConverter, setTitle, setWidth, setX, setY, show, showAndWait, showingProperty, titleProperty, widthProperty, xProperty, yProperty
-
Property Details
-
alertType
When creating an Alert instance, users must pass in anAlert.AlertType
enumeration value. It is by passing in this value that the Alert instance will configure itself appropriately (by setting default values for many of theDialog
properties, includingtitle
,header
, andgraphic
, as well as the defaultbuttons
that are expected in a dialog of the given type.- See Also:
-
-
Constructor Details
-
Alert
Creates an alert with the given AlertType (refer to theAlert.AlertType
documentation for clarification over which one is most appropriate).By passing in an AlertType, default values for the
title
,headerText
, andgraphic
properties are set, as well as the relevantbuttons
being installed. Once the Alert is instantiated, developers are able to modify the values of the alert as desired.It is important to note that the one property that does not have a default value set, and which therefore the developer must set, is the
content text
property (or alternatively, the developer may callalert.getDialogPane().setContent(Node)
if they want a more complex alert). If the contentText (or content) properties are not set, there is no useful information presented to end users.- Parameters:
alertType
- an alert with the given AlertType
-
Alert
Creates an alert with the given contentText, ButtonTypes, and AlertType (refer to theAlert.AlertType
documentation for clarification over which one is most appropriate).By passing in a variable number of ButtonType arguments, the developer is directly overriding the default buttons that will be displayed in the dialog, replacing the pre-defined buttons with whatever is specified in the varargs array.
By passing in an AlertType, default values for the
title
,headerText
, andgraphic
properties are set. Once the Alert is instantiated, developers are able to modify the values of the alert as desired.- Parameters:
alertType
- the alert typecontentText
- the content textbuttons
- the button types
-
-
Method Details
-
getAlertType
Gets the value of the property alertType.- Property description:
- When creating an Alert instance, users must pass in an
Alert.AlertType
enumeration value. It is by passing in this value that the Alert instance will configure itself appropriately (by setting default values for many of theDialog
properties, includingtitle
,header
, andgraphic
, as well as the defaultbuttons
that are expected in a dialog of the given type.
-
setAlertType
Sets the value of the property alertType.- Property description:
- When creating an Alert instance, users must pass in an
Alert.AlertType
enumeration value. It is by passing in this value that the Alert instance will configure itself appropriately (by setting default values for many of theDialog
properties, includingtitle
,header
, andgraphic
, as well as the defaultbuttons
that are expected in a dialog of the given type.
-
alertTypeProperty
When creating an Alert instance, users must pass in anAlert.AlertType
enumeration value. It is by passing in this value that the Alert instance will configure itself appropriately (by setting default values for many of theDialog
properties, includingtitle
,header
, andgraphic
, as well as the defaultbuttons
that are expected in a dialog of the given type.- See Also:
-
getButtonTypes
Returns anObservableList
of allButtonType
instances that are currently set inside this Alert instance. A ButtonType may either be one of the pre-defined types (e.g.ButtonType.OK
), or it may be a custom type (created via theButtonType(String)
orButtonType(String, javafx.scene.control.ButtonBar.ButtonData)
constructors.Readers should refer to the
ButtonType
class documentation for more details, but at a high level, each ButtonType instance is converted to a Node (although most commonly aButton
) via the (overridable)DialogPane.createButton(ButtonType)
method onDialogPane
.- Returns:
- an
ObservableList
of allButtonType
instances that are currently set inside this Alert instance
-