- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
public class Slider extends Control
The three fundamental variables of the slider are min
,
max
, and value
. The value
should always
be a number within the range defined by min
and
max
. min
should always be less than or equal to
max
(although a slider whose min
and
max
are equal is a degenerate case that makes no sense).
min
defaults to 0, whereas max
defaults to 100.
This first example creates a slider whose range, or span, goes from 0 to 1, and whose value defaults to .5:
Slider slider = new Slider(0, 1, 0.5);
This next example shows a slider with customized tick marks and tick mark labels, which also spans from 0 to 1:
Slider slider = new Slider(0, 1, 0.5); slider.setShowTickMarks(true); slider.setShowTickLabels(true); slider.setMajorTickUnit(0.25f); slider.setBlockIncrement(0.1f);
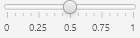
- Since:
- JavaFX 2.0
-
Property Summary
Properties Type Property Description DoubleProperty
blockIncrement
The amount by which to adjust the slider if the track of the slider is clicked.ObjectProperty<StringConverter<Double>>
labelFormatter
A function for formatting the label for a major tick.DoubleProperty
majorTickUnit
The unit distance between major tick marks.DoubleProperty
max
The maximum value represented by this Slider.IntegerProperty
minorTickCount
The number of minor ticks to place between any two major ticks.DoubleProperty
min
The minimum value represented by this Slider.ObjectProperty<Orientation>
orientation
The orientation of theSlider
can either be horizontal or vertical.BooleanProperty
showTickLabels
Indicates that the labels for tick marks should be shown.BooleanProperty
showTickMarks
Specifies whether theSkin
implementation should show tick marks.BooleanProperty
snapToTicks
Indicates whether thevalue
of theSlider
should always be aligned with the tick marks.BooleanProperty
valueChanging
When true, indicates the current value of this Slider is changing.DoubleProperty
value
The current value represented by this Slider.Properties declared in class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties declared in class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties declared in class javafx.scene.Parent
needsLayout
Properties declared in class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Field Summary
Fields declared in class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields declared in class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
-
Method Summary
Modifier and Type Method Description void
adjustValue(double newValue)
Adjustsvalue
to matchnewValue
.DoubleProperty
blockIncrementProperty()
The amount by which to adjust the slider if the track of the slider is clicked.void
decrement()
Decrements the value byblockIncrement
, bounded by max.double
getBlockIncrement()
Gets the value of the property blockIncrement.static List<CssMetaData<? extends Styleable,?>>
getClassCssMetaData()
protected List<CssMetaData<? extends Styleable,?>>
getControlCssMetaData()
StringConverter<Double>
getLabelFormatter()
Gets the value of the property labelFormatter.double
getMajorTickUnit()
Gets the value of the property majorTickUnit.double
getMax()
Gets the value of the property max.double
getMin()
Gets the value of the property min.int
getMinorTickCount()
Gets the value of the property minorTickCount.Orientation
getOrientation()
Gets the value of the property orientation.double
getValue()
Gets the value of the property value.void
increment()
Increments the value byblockIncrement
, bounded by max.boolean
isShowTickLabels()
Gets the value of the property showTickLabels.boolean
isShowTickMarks()
Gets the value of the property showTickMarks.boolean
isSnapToTicks()
Gets the value of the property snapToTicks.boolean
isValueChanging()
Gets the value of the property valueChanging.ObjectProperty<StringConverter<Double>>
labelFormatterProperty()
A function for formatting the label for a major tick.DoubleProperty
majorTickUnitProperty()
The unit distance between major tick marks.DoubleProperty
maxProperty()
The maximum value represented by this Slider.IntegerProperty
minorTickCountProperty()
The number of minor ticks to place between any two major ticks.DoubleProperty
minProperty()
The minimum value represented by this Slider.ObjectProperty<Orientation>
orientationProperty()
The orientation of theSlider
can either be horizontal or vertical.void
setBlockIncrement(double value)
Sets the value of the property blockIncrement.void
setLabelFormatter(StringConverter<Double> value)
Sets the value of the property labelFormatter.void
setMajorTickUnit(double value)
Sets the value of the property majorTickUnit.void
setMax(double value)
Sets the value of the property max.void
setMin(double value)
Sets the value of the property min.void
setMinorTickCount(int value)
Sets the value of the property minorTickCount.void
setOrientation(Orientation value)
Sets the value of the property orientation.void
setShowTickLabels(boolean value)
Sets the value of the property showTickLabels.void
setShowTickMarks(boolean value)
Sets the value of the property showTickMarks.void
setSnapToTicks(boolean value)
Sets the value of the property snapToTicks.void
setValue(double value)
Sets the value of the property value.void
setValueChanging(boolean value)
Sets the value of the property valueChanging.BooleanProperty
showTickLabelsProperty()
Indicates that the labels for tick marks should be shown.BooleanProperty
showTickMarksProperty()
Specifies whether theSkin
implementation should show tick marks.BooleanProperty
snapToTicksProperty()
Indicates whether thevalue
of theSlider
should always be aligned with the tick marks.BooleanProperty
valueChangingProperty()
When true, indicates the current value of this Slider is changing.DoubleProperty
valueProperty()
The current value represented by this Slider.Methods declared in class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, contextMenuProperty, createDefaultSkin, getContextMenu, getCssMetaData, getInitialFocusTraversable, getTooltip, isResizable, setContextMenu, setTooltip, skinProperty, tooltipProperty
Methods declared in class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, computePrefHeight, computePrefWidth, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods declared in class javafx.scene.Parent
getBaselineOffset, getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, layoutChildren, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods declared in class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode, getStyleClass
-
Property Details
-
max
The maximum value represented by this Slider. This must be a value greater thanmin
.- See Also:
getMax()
,setMax(double)
-
min
The minimum value represented by this Slider. This must be a value less thanmax
.- See Also:
getMin()
,setMin(double)
-
value
The current value represented by this Slider. This value must always be betweenmin
andmax
, inclusive. If it is ever out of bounds either due tomin
ormax
changing or due to itself being changed, then it will be clamped to always remain valid.- See Also:
getValue()
,setValue(double)
-
valueChanging
When true, indicates the current value of this Slider is changing. It provides notification that the value is changing. Once the value is computed, it is reset back to false.- See Also:
isValueChanging()
,setValueChanging(boolean)
-
orientation
The orientation of theSlider
can either be horizontal or vertical.- See Also:
getOrientation()
,setOrientation(Orientation)
-
showTickLabels
Indicates that the labels for tick marks should be shown. Typically aSkin
implementation will only show labels ifshowTickMarks
is also true.- See Also:
isShowTickLabels()
,setShowTickLabels(boolean)
-
showTickMarks
Specifies whether theSkin
implementation should show tick marks.- See Also:
isShowTickMarks()
,setShowTickMarks(boolean)
-
majorTickUnit
The unit distance between major tick marks. For example, if themin
is 0 and themax
is 100 and themajorTickUnit
is 25, then there would be 5 tick marks: one at position 0, one at position 25, one at position 50, one at position 75, and a final one at position 100.This value should be positive and should be a value less than the span. Out of range values are essentially the same as disabling tick marks.
- See Also:
getMajorTickUnit()
,setMajorTickUnit(double)
-
minorTickCount
The number of minor ticks to place between any two major ticks. This number should be positive or zero. Out of range values will disable disable minor ticks, as will a value of zero.- See Also:
getMinorTickCount()
,setMinorTickCount(int)
-
snapToTicks
Indicates whether thevalue
of theSlider
should always be aligned with the tick marks. This is honored even if the tick marks are not shown.- See Also:
isSnapToTicks()
,setSnapToTicks(boolean)
-
labelFormatter
A function for formatting the label for a major tick. The number representing the major tick will be passed to the function. If this function is not specified, then a default function will be used by theSkin
implementation. -
blockIncrement
The amount by which to adjust the slider if the track of the slider is clicked. This is used when manipulating the slider position using keys. IfsnapToTicks
is true then the nearest tick mark to the adjusted value will be used.- See Also:
getBlockIncrement()
,setBlockIncrement(double)
-
-
Constructor Details
-
Slider
public Slider()Creates a default Slider instance. -
Slider
public Slider(double min, double max, double value)Constructs a Slider control with the specified slider min, max and current value values.- Parameters:
min
- Slider minimum valuemax
- Slider maximum valuevalue
- Slider current value
-
-
Method Details
-
setMax
public final void setMax(double value)Sets the value of the property max.- Property description:
- The maximum value represented by this Slider. This must be a
value greater than
min
.
-
getMax
public final double getMax()Gets the value of the property max.- Property description:
- The maximum value represented by this Slider. This must be a
value greater than
min
.
-
maxProperty
The maximum value represented by this Slider. This must be a value greater thanmin
.- See Also:
getMax()
,setMax(double)
-
setMin
public final void setMin(double value)Sets the value of the property min.- Property description:
- The minimum value represented by this Slider. This must be a
value less than
max
.
-
getMin
public final double getMin()Gets the value of the property min.- Property description:
- The minimum value represented by this Slider. This must be a
value less than
max
.
-
minProperty
The minimum value represented by this Slider. This must be a value less thanmax
.- See Also:
getMin()
,setMin(double)
-
setValue
public final void setValue(double value)Sets the value of the property value. -
getValue
public final double getValue()Gets the value of the property value. -
valueProperty
The current value represented by this Slider. This value must always be betweenmin
andmax
, inclusive. If it is ever out of bounds either due tomin
ormax
changing or due to itself being changed, then it will be clamped to always remain valid.- See Also:
getValue()
,setValue(double)
-
setValueChanging
public final void setValueChanging(boolean value)Sets the value of the property valueChanging.- Property description:
- When true, indicates the current value of this Slider is changing. It provides notification that the value is changing. Once the value is computed, it is reset back to false.
-
isValueChanging
public final boolean isValueChanging()Gets the value of the property valueChanging.- Property description:
- When true, indicates the current value of this Slider is changing. It provides notification that the value is changing. Once the value is computed, it is reset back to false.
-
valueChangingProperty
When true, indicates the current value of this Slider is changing. It provides notification that the value is changing. Once the value is computed, it is reset back to false.- See Also:
isValueChanging()
,setValueChanging(boolean)
-
setOrientation
Sets the value of the property orientation.- Property description:
- The orientation of the
Slider
can either be horizontal or vertical.
-
getOrientation
Gets the value of the property orientation.- Property description:
- The orientation of the
Slider
can either be horizontal or vertical.
-
orientationProperty
The orientation of theSlider
can either be horizontal or vertical.- See Also:
getOrientation()
,setOrientation(Orientation)
-
setShowTickLabels
public final void setShowTickLabels(boolean value)Sets the value of the property showTickLabels.- Property description:
- Indicates that the labels for tick marks should be shown. Typically a
Skin
implementation will only show labels ifshowTickMarks
is also true.
-
isShowTickLabels
public final boolean isShowTickLabels()Gets the value of the property showTickLabels.- Property description:
- Indicates that the labels for tick marks should be shown. Typically a
Skin
implementation will only show labels ifshowTickMarks
is also true.
-
showTickLabelsProperty
Indicates that the labels for tick marks should be shown. Typically aSkin
implementation will only show labels ifshowTickMarks
is also true.- See Also:
isShowTickLabels()
,setShowTickLabels(boolean)
-
setShowTickMarks
public final void setShowTickMarks(boolean value)Sets the value of the property showTickMarks.- Property description:
- Specifies whether the
Skin
implementation should show tick marks.
-
isShowTickMarks
public final boolean isShowTickMarks()Gets the value of the property showTickMarks.- Property description:
- Specifies whether the
Skin
implementation should show tick marks.
-
showTickMarksProperty
Specifies whether theSkin
implementation should show tick marks.- See Also:
isShowTickMarks()
,setShowTickMarks(boolean)
-
setMajorTickUnit
public final void setMajorTickUnit(double value)Sets the value of the property majorTickUnit.- Property description:
- The unit distance between major tick marks. For example, if
the
min
is 0 and themax
is 100 and themajorTickUnit
is 25, then there would be 5 tick marks: one at position 0, one at position 25, one at position 50, one at position 75, and a final one at position 100.This value should be positive and should be a value less than the span. Out of range values are essentially the same as disabling tick marks.
-
getMajorTickUnit
public final double getMajorTickUnit()Gets the value of the property majorTickUnit.- Property description:
- The unit distance between major tick marks. For example, if
the
min
is 0 and themax
is 100 and themajorTickUnit
is 25, then there would be 5 tick marks: one at position 0, one at position 25, one at position 50, one at position 75, and a final one at position 100.This value should be positive and should be a value less than the span. Out of range values are essentially the same as disabling tick marks.
-
majorTickUnitProperty
The unit distance between major tick marks. For example, if themin
is 0 and themax
is 100 and themajorTickUnit
is 25, then there would be 5 tick marks: one at position 0, one at position 25, one at position 50, one at position 75, and a final one at position 100.This value should be positive and should be a value less than the span. Out of range values are essentially the same as disabling tick marks.
- See Also:
getMajorTickUnit()
,setMajorTickUnit(double)
-
setMinorTickCount
public final void setMinorTickCount(int value)Sets the value of the property minorTickCount.- Property description:
- The number of minor ticks to place between any two major ticks. This number should be positive or zero. Out of range values will disable disable minor ticks, as will a value of zero.
-
getMinorTickCount
public final int getMinorTickCount()Gets the value of the property minorTickCount.- Property description:
- The number of minor ticks to place between any two major ticks. This number should be positive or zero. Out of range values will disable disable minor ticks, as will a value of zero.
-
minorTickCountProperty
The number of minor ticks to place between any two major ticks. This number should be positive or zero. Out of range values will disable disable minor ticks, as will a value of zero.- See Also:
getMinorTickCount()
,setMinorTickCount(int)
-
setSnapToTicks
public final void setSnapToTicks(boolean value)Sets the value of the property snapToTicks.- Property description:
- Indicates whether the
value
of theSlider
should always be aligned with the tick marks. This is honored even if the tick marks are not shown.
-
isSnapToTicks
public final boolean isSnapToTicks()Gets the value of the property snapToTicks.- Property description:
- Indicates whether the
value
of theSlider
should always be aligned with the tick marks. This is honored even if the tick marks are not shown.
-
snapToTicksProperty
Indicates whether thevalue
of theSlider
should always be aligned with the tick marks. This is honored even if the tick marks are not shown.- See Also:
isSnapToTicks()
,setSnapToTicks(boolean)
-
setLabelFormatter
Sets the value of the property labelFormatter.- Property description:
- A function for formatting the label for a major tick. The number
representing the major tick will be passed to the function. If this
function is not specified, then a default function will be used by
the
Skin
implementation.
-
getLabelFormatter
Gets the value of the property labelFormatter.- Property description:
- A function for formatting the label for a major tick. The number
representing the major tick will be passed to the function. If this
function is not specified, then a default function will be used by
the
Skin
implementation.
-
labelFormatterProperty
A function for formatting the label for a major tick. The number representing the major tick will be passed to the function. If this function is not specified, then a default function will be used by theSkin
implementation. -
setBlockIncrement
public final void setBlockIncrement(double value)Sets the value of the property blockIncrement.- Property description:
- The amount by which to adjust the slider if the track of the slider is
clicked. This is used when manipulating the slider position using keys. If
snapToTicks
is true then the nearest tick mark to the adjusted value will be used.
-
getBlockIncrement
public final double getBlockIncrement()Gets the value of the property blockIncrement.- Property description:
- The amount by which to adjust the slider if the track of the slider is
clicked. This is used when manipulating the slider position using keys. If
snapToTicks
is true then the nearest tick mark to the adjusted value will be used.
-
blockIncrementProperty
The amount by which to adjust the slider if the track of the slider is clicked. This is used when manipulating the slider position using keys. IfsnapToTicks
is true then the nearest tick mark to the adjusted value will be used.- See Also:
getBlockIncrement()
,setBlockIncrement(double)
-
adjustValue
public void adjustValue(double newValue)Adjustsvalue
to matchnewValue
. Thevalue
is the actual amount between themin
andmax
. This function also takes into accountsnapToTicks
, which is the main difference between adjustValue and setValue. It also ensures that the value is some valid number between min and max. Note: This function is intended to be used by experts, primarily by those implementing new Skins or Behaviors. It is not common for developers or designers to access this function directly.- Parameters:
newValue
- the new adjusted value
-
increment
public void increment()Increments the value byblockIncrement
, bounded by max. If the max is less than or equal to the min, then this method does nothing. -
decrement
public void decrement()Decrements the value byblockIncrement
, bounded by max. If the max is less than or equal to the min, then this method does nothing. -
getClassCssMetaData
- Returns:
- The CssMetaData associated with this class, which may include the CssMetaData of its superclasses.
- Since:
- JavaFX 8.0
-
getControlCssMetaData
- Overrides:
getControlCssMetaData
in classControl
- Returns:
- unmodifiable list of the controls css styleable properties
- Since:
- JavaFX 8.0
-