java.lang.Object
javafx.scene.Node
javafx.scene.media.MediaView
- All Implemented Interfaces:
Styleable
,EventTarget
public class MediaView extends Node
A
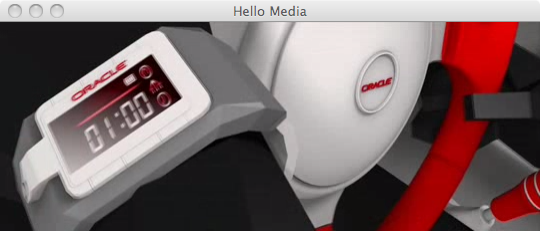
Node
that provides a view of Media
being played by a
MediaPlayer
.
The following code snippet provides a simple example of an
Application.start()
method which displays a video:
public void start(Stage stage) {
// Create and set the Scene.
Scene scene = new Scene(new Group(), 540, 209);
stage.setScene(scene);
// Name and display the Stage.
stage.setTitle("Hello Media");
stage.show();
// Create the media source.
String source = getParameters().getRaw().get(0);
Media media = new Media(source);
// Create the player and set to play automatically.
MediaPlayer mediaPlayer = new MediaPlayer(media);
mediaPlayer.setAutoPlay(true);
// Create the view and add it to the Scene.
MediaView mediaView = new MediaView(mediaPlayer);
((Group) scene.getRoot()).getChildren().add(mediaView);
}
The foregoing code will display the video as:
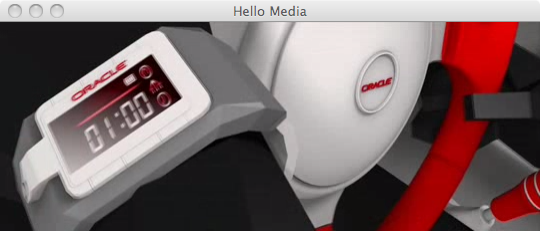
- Since:
- JavaFX 2.0
-
Property Summary
Properties Type Property Description DoubleProperty
fitHeight
Determines the height of the bounding box within which the source media is resized as necessary to fit.DoubleProperty
fitWidth
Determines the width of the bounding box within which the source media is resized as necessary to fit.ObjectProperty<MediaPlayer>
mediaPlayer
ThemediaPlayer
whose output will be handled by this view.ObjectProperty<EventHandler<MediaErrorEvent>>
onError
Event handler to be invoked whenever an error occurs on thisMediaView
.BooleanProperty
preserveRatio
Whether to preserve the aspect ratio (width / height) of the media when scaling it to fit the node.BooleanProperty
smooth
If set totrue
a better quality filtering algorithm will be used when scaling this video to fit within the bounding box provided byfitWidth
andfitHeight
or when transforming.ObjectProperty<Rectangle2D>
viewport
Specifies a rectangular viewport into the media frame.DoubleProperty
x
Defines the current x coordinate of theMediaView
origin.DoubleProperty
y
Defines the current y coordinate of theMediaView
origin.Properties inherited from class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Field Summary
-
Constructor Summary
Constructors Constructor Description MediaView()
Creates aMediaView
instance with no associatedMediaPlayer
.MediaView(MediaPlayer mediaPlayer)
Creates aMediaView
instance associated with the specifiedMediaPlayer
. -
Method Summary
Modifier and Type Method Description DoubleProperty
fitHeightProperty()
Determines the height of the bounding box within which the source media is resized as necessary to fit.DoubleProperty
fitWidthProperty()
Determines the width of the bounding box within which the source media is resized as necessary to fit.double
getFitHeight()
Retrieves the height of the bounding box of the resized media.double
getFitWidth()
Retrieves the width of the bounding box of the resized media.MediaPlayer
getMediaPlayer()
Retrieves theMediaPlayer
whose output is being handled by this view.EventHandler<MediaErrorEvent>
getOnError()
Retrieves the error event handler.Rectangle2D
getViewport()
Retrieves the rectangular viewport into the media frame.double
getX()
Retrieves the x coordinate of theMediaView
origin.double
getY()
Retrieves the y coordinate of theMediaView
origin.boolean
isPreserveRatio()
Returns whether the media aspect ratio is preserved when scaling.boolean
isSmooth()
Returns whether to smooth the media when scaling.ObjectProperty<MediaPlayer>
mediaPlayerProperty()
ThemediaPlayer
whose output will be handled by this view.ObjectProperty<EventHandler<MediaErrorEvent>>
onErrorProperty()
Event handler to be invoked whenever an error occurs on thisMediaView
.BooleanProperty
preserveRatioProperty()
Whether to preserve the aspect ratio (width / height) of the media when scaling it to fit the node.void
setFitHeight(double value)
Sets the height of the bounding box of the resized media.void
setFitWidth(double value)
Sets the width of the bounding box of the resized media.void
setMediaPlayer(MediaPlayer value)
Sets theMediaPlayer
whose output will be handled by this view.void
setOnError(EventHandler<MediaErrorEvent> value)
Sets the error event handler.void
setPreserveRatio(boolean value)
Sets whether to preserve the media aspect ratio when scaling.void
setSmooth(boolean value)
Sets whether to smooth the media when scaling.void
setViewport(Rectangle2D value)
Sets the rectangular viewport into the media frame.void
setX(double value)
Sets the x coordinate of theMediaView
origin.void
setY(double value)
Sets the y coordinate of theMediaView
origin.BooleanProperty
smoothProperty()
If set totrue
a better quality filtering algorithm will be used when scaling this video to fit within the bounding box provided byfitWidth
andfitHeight
or when transforming.ObjectProperty<Rectangle2D>
viewportProperty()
Specifies a rectangular viewport into the media frame.DoubleProperty
xProperty()
Defines the current x coordinate of theMediaView
origin.DoubleProperty
yProperty()
Defines the current y coordinate of theMediaView
origin.Methods inherited from class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBaselineOffset, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClassCssMetaData, getClip, getContentBias, getCssMetaData, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInitialFocusTraversable, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isResizable, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, maxHeight, maxWidth, minHeight, minWidth, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, prefHeight, prefWidth, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, resize, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
-
Property Details
-
mediaPlayer
ThemediaPlayer
whose output will be handled by this view. Setting this value does not affect the status of theMediaPlayer
, e.g., if theMediaPlayer
was playing prior to settingmediaPlayer
then it will continue playing.- See Also:
getMediaPlayer()
,setMediaPlayer(MediaPlayer)
-
onError
Event handler to be invoked whenever an error occurs on thisMediaView
.- See Also:
getOnError()
,setOnError(EventHandler)
-
preserveRatio
Whether to preserve the aspect ratio (width / height) of the media when scaling it to fit the node. If the aspect ratio is not preserved, the media will be stretched or sheared in both dimensions to fit the dimensions of the node. The default value istrue
.- See Also:
isPreserveRatio()
,setPreserveRatio(boolean)
-
smooth
If set totrue
a better quality filtering algorithm will be used when scaling this video to fit within the bounding box provided byfitWidth
andfitHeight
or when transforming. If set tofalse
a faster but lesser quality filtering will be used. The default value depends on platform configuration.- See Also:
isSmooth()
,setSmooth(boolean)
-
x
Defines the current x coordinate of theMediaView
origin.- See Also:
getX()
,setX(double)
-
y
Defines the current y coordinate of theMediaView
origin.- See Also:
getY()
,setY(double)
-
fitWidth
Determines the width of the bounding box within which the source media is resized as necessary to fit. Ifvalue ≤ 0
, then the width of the bounding box will be set to the natural width of the media, butfitWidth
will be set to the supplied parameter, even if non-positive.See
preserveRatio
for information on interaction between media viewsfitWidth
,fitHeight
andpreserveRatio
attributes.- See Also:
getFitWidth()
,setFitWidth(double)
-
fitHeight
Determines the height of the bounding box within which the source media is resized as necessary to fit. Ifvalue ≤ 0
, then the height of the bounding box will be set to the natural height of the media, butfitHeight
will be set to the supplied parameter, even if non-positive.See
preserveRatio
for information on interaction between media viewsfitWidth
,fitHeight
andpreserveRatio
attributes.- See Also:
getFitHeight()
,setFitHeight(double)
-
viewport
Specifies a rectangular viewport into the media frame. The viewport is a rectangle specified in the coordinates of the media frame. The resulting bounds prior to scaling will be the size of the viewport. The displayed image will include the intersection of the frame and the viewport. The viewport can exceed the size of the frame, but only the intersection will be displayed. Settingviewport
to null will clear the viewport.- See Also:
getViewport()
,setViewport(Rectangle2D)
-
-
Constructor Details
-
MediaView
public MediaView()Creates aMediaView
instance with no associatedMediaPlayer
. -
MediaView
Creates aMediaView
instance associated with the specifiedMediaPlayer
. Equivalent toMediaPlayer player; // initialization omitted MediaView view = new MediaView(); view.setMediaPlayer(player);
- Parameters:
mediaPlayer
- theMediaPlayer
the playback of which is to be viewed via this class.
-
-
Method Details
-
setMediaPlayer
Sets theMediaPlayer
whose output will be handled by this view.- Parameters:
value
- the associatedMediaPlayer
.
-
getMediaPlayer
Retrieves theMediaPlayer
whose output is being handled by this view.- Returns:
- the associated
MediaPlayer
.
-
mediaPlayerProperty
ThemediaPlayer
whose output will be handled by this view. Setting this value does not affect the status of theMediaPlayer
, e.g., if theMediaPlayer
was playing prior to settingmediaPlayer
then it will continue playing.- See Also:
getMediaPlayer()
,setMediaPlayer(MediaPlayer)
-
setOnError
Sets the error event handler.- Parameters:
value
- the error event handler.
-
getOnError
Retrieves the error event handler.- Returns:
- the error event handler.
-
onErrorProperty
Event handler to be invoked whenever an error occurs on thisMediaView
.- See Also:
getOnError()
,setOnError(EventHandler)
-
setPreserveRatio
public final void setPreserveRatio(boolean value)Sets whether to preserve the media aspect ratio when scaling.- Parameters:
value
- whether to preserve the media aspect ratio.
-
isPreserveRatio
public final boolean isPreserveRatio()Returns whether the media aspect ratio is preserved when scaling.- Returns:
- whether the media aspect ratio is preserved.
-
preserveRatioProperty
Whether to preserve the aspect ratio (width / height) of the media when scaling it to fit the node. If the aspect ratio is not preserved, the media will be stretched or sheared in both dimensions to fit the dimensions of the node. The default value istrue
.- See Also:
isPreserveRatio()
,setPreserveRatio(boolean)
-
setSmooth
public final void setSmooth(boolean value)Sets whether to smooth the media when scaling.- Parameters:
value
- whether to smooth the media.
-
isSmooth
public final boolean isSmooth()Returns whether to smooth the media when scaling.- Returns:
- whether to smooth the media
-
smoothProperty
If set totrue
a better quality filtering algorithm will be used when scaling this video to fit within the bounding box provided byfitWidth
andfitHeight
or when transforming. If set tofalse
a faster but lesser quality filtering will be used. The default value depends on platform configuration.- See Also:
isSmooth()
,setSmooth(boolean)
-
setX
public final void setX(double value)Sets the x coordinate of theMediaView
origin.- Parameters:
value
- the x coordinate of the origin of the view.
-
getX
public final double getX()Retrieves the x coordinate of theMediaView
origin.- Returns:
- the x coordinate of the origin of the view.
-
xProperty
Defines the current x coordinate of theMediaView
origin.- See Also:
getX()
,setX(double)
-
setY
public final void setY(double value)Sets the y coordinate of theMediaView
origin.- Parameters:
value
- the y coordinate of the origin of the view.
-
getY
public final double getY()Retrieves the y coordinate of theMediaView
origin.- Returns:
- the y coordinate of the origin of the view.
-
yProperty
Defines the current y coordinate of theMediaView
origin.- See Also:
getY()
,setY(double)
-
setFitWidth
public final void setFitWidth(double value)Sets the width of the bounding box of the resized media.- Parameters:
value
- the width of the resized media.
-
getFitWidth
public final double getFitWidth()Retrieves the width of the bounding box of the resized media.- Returns:
- the height of the resized media.
-
fitWidthProperty
Determines the width of the bounding box within which the source media is resized as necessary to fit. Ifvalue ≤ 0
, then the width of the bounding box will be set to the natural width of the media, butfitWidth
will be set to the supplied parameter, even if non-positive.See
preserveRatio
for information on interaction between media viewsfitWidth
,fitHeight
andpreserveRatio
attributes.- See Also:
getFitWidth()
,setFitWidth(double)
-
setFitHeight
public final void setFitHeight(double value)Sets the height of the bounding box of the resized media.- Parameters:
value
- the height of the resized media.
-
getFitHeight
public final double getFitHeight()Retrieves the height of the bounding box of the resized media.- Returns:
- the height of the resized media.
-
fitHeightProperty
Determines the height of the bounding box within which the source media is resized as necessary to fit. Ifvalue ≤ 0
, then the height of the bounding box will be set to the natural height of the media, butfitHeight
will be set to the supplied parameter, even if non-positive.See
preserveRatio
for information on interaction between media viewsfitWidth
,fitHeight
andpreserveRatio
attributes.- See Also:
getFitHeight()
,setFitHeight(double)
-
setViewport
Sets the rectangular viewport into the media frame.- Parameters:
value
- the rectangular viewport.
-
getViewport
Retrieves the rectangular viewport into the media frame.- Returns:
- the rectangular viewport.
-
viewportProperty
Specifies a rectangular viewport into the media frame. The viewport is a rectangle specified in the coordinates of the media frame. The resulting bounds prior to scaling will be the size of the viewport. The displayed image will include the intersection of the frame and the viewport. The viewport can exceed the size of the frame, but only the intersection will be displayed. Settingviewport
to null will clear the viewport.- See Also:
getViewport()
,setViewport(Rectangle2D)
-