- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
Tabs
. Only one tab
is visible at a time. Tabs are added to the TabPane by using the getTabs()
.
Tabs in a TabPane can be positioned at any of the four sides by specifying the
Side
.
A TabPane has two modes floating or recessed. Applying the styleclass STYLE_CLASS_FLOATING will change the TabPane mode to floating.
The tabs width and height can be set to a specific size by setting the min and max for height and width. TabPane default width will be determined by the largest content width in the TabPane. This is the same for the height. If a different size is desired the width and height of the TabPane can be overridden by setting the min, pref and max size.
When the number of tabs do not fit the TabPane a menu button will appear on the right. The menu button is used to select the tabs that are currently not visible.
Example:
TabPane tabPane = new TabPane();
Tab tab = new Tab();
tab.setText("new tab");
tab.setContent(new Rectangle(100, 50, Color.LIGHTSTEELBLUE));
tabPane.getTabs().add(tab);
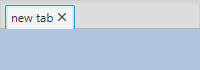
- Since:
- JavaFX 2.0
- See Also:
-
Property Summary
PropertiesTypePropertyDescriptionfinal BooleanProperty
Specifies whether the graphic inside aTab
is rotated or not, such that it is always upright, or rotated in the same way as theTab
text is.final ObjectProperty<SingleSelectionModel<Tab>>
The selection model used for selecting tabs.final ObjectProperty<Side>
The position to place the tabs in thisTabPane
.Specifies how theTabPane
handles tab closing from an end-user's perspective.The drag policy for the tabs.final DoubleProperty
The maximum height of aTab
in theTabPane
.final DoubleProperty
The maximum width of aTab
in theTabPane
.final DoubleProperty
The minimum height of aTab
in theTabPane
.final DoubleProperty
The minimum width of aTab
in theTabPane
.Properties declared in class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties declared in class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties declared in class javafx.scene.Parent
needsLayout
Properties declared in class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Specifies how theTabPane
handles tab closing from an end-user's perspective.static enum
This enum specifies drag policies for tabs in a TabPane. -
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final String
TabPane mode will be changed to floating allowing the TabPane to be placed alongside other control.Fields declared in class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields declared in class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionstatic List<CssMetaData<? extends Styleable,
?>> Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.List<CssMetaData<? extends Styleable,
?>> Gets the unmodifiable list of the control's CSS-styleable properties.final SingleSelectionModel<Tab>
Gets the value of the property selectionModel.final Side
getSide()
Gets the value of the property side.final TabPane.TabClosingPolicy
Gets the value of the property tabClosingPolicy.final TabPane.TabDragPolicy
Gets the value of the property tabDragPolicy.final double
Gets the value of the property tabMaxHeight.final double
Gets the value of the property tabMaxWidth.final double
Gets the value of the property tabMinHeight.final double
Gets the value of the property tabMinWidth.final ObservableList<Tab>
getTabs()
The tabs to display in this TabPane.final boolean
Gets the value of the property rotateGraphic.final BooleanProperty
Specifies whether the graphic inside aTab
is rotated or not, such that it is always upright, or rotated in the same way as theTab
text is.final ObjectProperty<SingleSelectionModel<Tab>>
The selection model used for selecting tabs.final void
setRotateGraphic
(boolean value) Sets the value of the property rotateGraphic.final void
setSelectionModel
(SingleSelectionModel<Tab> value) Sets the value of the property selectionModel.final void
Sets the value of the property side.final void
Sets the value of the property tabClosingPolicy.final void
Sets the value of the property tabDragPolicy.final void
setTabMaxHeight
(double value) Sets the value of the property tabMaxHeight.final void
setTabMaxWidth
(double value) Sets the value of the property tabMaxWidth.final void
setTabMinHeight
(double value) Sets the value of the property tabMinHeight.final void
setTabMinWidth
(double value) Sets the value of the property tabMinWidth.final ObjectProperty<Side>
The position to place the tabs in thisTabPane
.Specifies how theTabPane
handles tab closing from an end-user's perspective.The drag policy for the tabs.final DoubleProperty
The maximum height of aTab
in theTabPane
.final DoubleProperty
The maximum width of aTab
in theTabPane
.final DoubleProperty
The minimum height of aTab
in theTabPane
.final DoubleProperty
The minimum width of aTab
in theTabPane
.Methods declared in class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, contextMenuProperty, createDefaultSkin, getContextMenu, getCssMetaData, getInitialFocusTraversable, getSkin, getTooltip, isResizable, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods declared in class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, computePrefHeight, computePrefWidth, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods declared in class javafx.scene.Parent
getBaselineOffset, getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, layoutChildren, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods declared in class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
selectionModel
The selection model used for selecting tabs. Changing the model alters how the tabs are selected and which tabs are first or last. -
side
The position to place the tabs in thisTabPane
. Changes to the value of this property immediately updates the location of the tabs.- Default value:
Side.Top
- See Also:
-
tabClosingPolicy
Specifies how theTabPane
handles tab closing from an end-user's perspective.Refer to the
TabPane.TabClosingPolicy
enumeration for further details.- Default value:
TabClosingPolicy.SELECTED_TAB
- See Also:
-
rotateGraphic
Specifies whether the graphic inside aTab
is rotated or not, such that it is always upright, or rotated in the same way as theTab
text is.If the value is
false
, the graphic isn't rotated, resulting in it always appearing upright. If the value istrue
, the graphic is rotated with theTab
text.- Default value:
false
- See Also:
-
tabMinWidth
The minimum width of aTab
in theTabPane
. This can be used to limit the length of text in tabs to prevent truncation. Setting the same minimum and maximum widths will fix the width of theTab
.This value can also be set via CSS using
-fx-tab-min-width
.- Default value:
- 0
- See Also:
-
tabMaxWidth
The maximum width of aTab
in theTabPane
. This can be used to limit the length of text in tabs to prevent truncation. If theTab
text is longer than the maximum width, the text will be truncated. Setting the same minimum and maximum widths will fix the width of theTab
.This value can also be set via CSS using
-fx-tab-max-width
.- Default value:
Double.MAX_VALUE
- See Also:
-
tabMinHeight
The minimum height of aTab
in theTabPane
. This can be used to limit the height of tabs. Setting the same minimum and maximum heights will fix the height of theTab
.This value can also be set via CSS using
-fx-tab-min-height
.- Default value:
- 0
- See Also:
-
tabMaxHeight
The maximum height of aTab
in theTabPane
. This can be used to limit the height of tabs. Setting the same minimum and maximum heights will fix the height of theTab
.This value can also be set via CSS using
-fx-tab-max-height
.- Default value:
Double.MAX_VALUE
- See Also:
-
tabDragPolicy
The drag policy for the tabs. It specifies if tabs can be reordered or not.- Default value:
TabDragPolicy.FIXED
- Since:
- 10
- See Also:
-
-
Field Details
-
STYLE_CLASS_FLOATING
TabPane mode will be changed to floating allowing the TabPane to be placed alongside other control.- See Also:
-
-
Constructor Details
-
TabPane
public TabPane()Constructs a new TabPane. -
TabPane
Constructs a new TabPane with the given tabs set to show.- Parameters:
tabs
- Thetabs
to display inside the TabPane.- Since:
- JavaFX 8u40
-
-
Method Details
-
getTabs
The tabs to display in this TabPane. Changing this ObservableList will immediately result in the TabPane updating to display the new contents of this ObservableList.If the tabs ObservableList changes, the selected tab will remain the previously selected tab, if it remains within this ObservableList. If the previously selected tab is no longer in the tabs ObservableList, the selected tab will become the first tab in the ObservableList.
- Returns:
- the list of tabs
-
setSelectionModel
Sets the value of the property selectionModel.- Property description:
- The selection model used for selecting tabs. Changing the model alters how the tabs are selected and which tabs are first or last.
-
getSelectionModel
Gets the value of the property selectionModel.- Property description:
- The selection model used for selecting tabs. Changing the model alters how the tabs are selected and which tabs are first or last.
-
selectionModelProperty
The selection model used for selecting tabs. Changing the model alters how the tabs are selected and which tabs are first or last. -
setSide
Sets the value of the property side.- Property description:
- The position to place the tabs in this
TabPane
. Changes to the value of this property immediately updates the location of the tabs. - Default value:
Side.Top
-
getSide
Gets the value of the property side.- Property description:
- The position to place the tabs in this
TabPane
. Changes to the value of this property immediately updates the location of the tabs. - Default value:
Side.Top
-
sideProperty
The position to place the tabs in thisTabPane
. Changes to the value of this property immediately updates the location of the tabs.- Default value:
Side.Top
- See Also:
-
setTabClosingPolicy
Sets the value of the property tabClosingPolicy.- Property description:
- Specifies how the
TabPane
handles tab closing from an end-user's perspective.Refer to the
TabPane.TabClosingPolicy
enumeration for further details. - Default value:
TabClosingPolicy.SELECTED_TAB
-
getTabClosingPolicy
Gets the value of the property tabClosingPolicy.- Property description:
- Specifies how the
TabPane
handles tab closing from an end-user's perspective.Refer to the
TabPane.TabClosingPolicy
enumeration for further details. - Default value:
TabClosingPolicy.SELECTED_TAB
-
tabClosingPolicyProperty
Specifies how theTabPane
handles tab closing from an end-user's perspective.Refer to the
TabPane.TabClosingPolicy
enumeration for further details.- Default value:
TabClosingPolicy.SELECTED_TAB
- See Also:
-
setRotateGraphic
public final void setRotateGraphic(boolean value) Sets the value of the property rotateGraphic.- Property description:
- Specifies whether the graphic inside a
Tab
is rotated or not, such that it is always upright, or rotated in the same way as theTab
text is.If the value is
false
, the graphic isn't rotated, resulting in it always appearing upright. If the value istrue
, the graphic is rotated with theTab
text. - Default value:
false
-
isRotateGraphic
public final boolean isRotateGraphic()Gets the value of the property rotateGraphic.- Property description:
- Specifies whether the graphic inside a
Tab
is rotated or not, such that it is always upright, or rotated in the same way as theTab
text is.If the value is
false
, the graphic isn't rotated, resulting in it always appearing upright. If the value istrue
, the graphic is rotated with theTab
text. - Default value:
false
-
rotateGraphicProperty
Specifies whether the graphic inside aTab
is rotated or not, such that it is always upright, or rotated in the same way as theTab
text is.If the value is
false
, the graphic isn't rotated, resulting in it always appearing upright. If the value istrue
, the graphic is rotated with theTab
text.- Default value:
false
- See Also:
-
setTabMinWidth
public final void setTabMinWidth(double value) Sets the value of the property tabMinWidth.- Property description:
- The minimum width of a
Tab
in theTabPane
. This can be used to limit the length of text in tabs to prevent truncation. Setting the same minimum and maximum widths will fix the width of theTab
.This value can also be set via CSS using
-fx-tab-min-width
. - Default value:
- 0
-
getTabMinWidth
public final double getTabMinWidth()Gets the value of the property tabMinWidth.- Property description:
- The minimum width of a
Tab
in theTabPane
. This can be used to limit the length of text in tabs to prevent truncation. Setting the same minimum and maximum widths will fix the width of theTab
.This value can also be set via CSS using
-fx-tab-min-width
. - Default value:
- 0
-
tabMinWidthProperty
The minimum width of aTab
in theTabPane
. This can be used to limit the length of text in tabs to prevent truncation. Setting the same minimum and maximum widths will fix the width of theTab
.This value can also be set via CSS using
-fx-tab-min-width
.- Default value:
- 0
- See Also:
-
setTabMaxWidth
public final void setTabMaxWidth(double value) Sets the value of the property tabMaxWidth.- Property description:
- The maximum width of a
Tab
in theTabPane
. This can be used to limit the length of text in tabs to prevent truncation. If theTab
text is longer than the maximum width, the text will be truncated. Setting the same minimum and maximum widths will fix the width of theTab
.This value can also be set via CSS using
-fx-tab-max-width
. - Default value:
Double.MAX_VALUE
-
getTabMaxWidth
public final double getTabMaxWidth()Gets the value of the property tabMaxWidth.- Property description:
- The maximum width of a
Tab
in theTabPane
. This can be used to limit the length of text in tabs to prevent truncation. If theTab
text is longer than the maximum width, the text will be truncated. Setting the same minimum and maximum widths will fix the width of theTab
.This value can also be set via CSS using
-fx-tab-max-width
. - Default value:
Double.MAX_VALUE
-
tabMaxWidthProperty
The maximum width of aTab
in theTabPane
. This can be used to limit the length of text in tabs to prevent truncation. If theTab
text is longer than the maximum width, the text will be truncated. Setting the same minimum and maximum widths will fix the width of theTab
.This value can also be set via CSS using
-fx-tab-max-width
.- Default value:
Double.MAX_VALUE
- See Also:
-
setTabMinHeight
public final void setTabMinHeight(double value) Sets the value of the property tabMinHeight.- Property description:
- The minimum height of a
Tab
in theTabPane
. This can be used to limit the height of tabs. Setting the same minimum and maximum heights will fix the height of theTab
.This value can also be set via CSS using
-fx-tab-min-height
. - Default value:
- 0
-
getTabMinHeight
public final double getTabMinHeight()Gets the value of the property tabMinHeight.- Property description:
- The minimum height of a
Tab
in theTabPane
. This can be used to limit the height of tabs. Setting the same minimum and maximum heights will fix the height of theTab
.This value can also be set via CSS using
-fx-tab-min-height
. - Default value:
- 0
-
tabMinHeightProperty
The minimum height of aTab
in theTabPane
. This can be used to limit the height of tabs. Setting the same minimum and maximum heights will fix the height of theTab
.This value can also be set via CSS using
-fx-tab-min-height
.- Default value:
- 0
- See Also:
-
setTabMaxHeight
public final void setTabMaxHeight(double value) Sets the value of the property tabMaxHeight.- Property description:
- The maximum height of a
Tab
in theTabPane
. This can be used to limit the height of tabs. Setting the same minimum and maximum heights will fix the height of theTab
.This value can also be set via CSS using
-fx-tab-max-height
. - Default value:
Double.MAX_VALUE
-
getTabMaxHeight
public final double getTabMaxHeight()Gets the value of the property tabMaxHeight.- Property description:
- The maximum height of a
Tab
in theTabPane
. This can be used to limit the height of tabs. Setting the same minimum and maximum heights will fix the height of theTab
.This value can also be set via CSS using
-fx-tab-max-height
. - Default value:
Double.MAX_VALUE
-
tabMaxHeightProperty
The maximum height of aTab
in theTabPane
. This can be used to limit the height of tabs. Setting the same minimum and maximum heights will fix the height of theTab
.This value can also be set via CSS using
-fx-tab-max-height
.- Default value:
Double.MAX_VALUE
- See Also:
-
getClassCssMetaData
Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.- Returns:
- the
CssMetaData
- Since:
- JavaFX 8.0
-
getControlCssMetaData
Gets the unmodifiable list of the control's CSS-styleable properties.- Overrides:
getControlCssMetaData
in classControl
- Returns:
- the unmodifiable list of the control's CSS-styleable properties
- Since:
- JavaFX 8.0
-
tabDragPolicyProperty
The drag policy for the tabs. It specifies if tabs can be reordered or not.- Default value:
TabDragPolicy.FIXED
- Since:
- 10
- See Also:
-
setTabDragPolicy
Sets the value of the property tabDragPolicy.- Property description:
- The drag policy for the tabs. It specifies if tabs can be reordered or not.
- Default value:
TabDragPolicy.FIXED
- Since:
- 10
-
getTabDragPolicy
Gets the value of the property tabDragPolicy.- Property description:
- The drag policy for the tabs. It specifies if tabs can be reordered or not.
- Default value:
TabDragPolicy.FIXED
- Since:
- 10
-