- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
@DefaultProperty("tabs") public class TabPane extends Control
A control that allows switching between a group of Tabs
. Only one tab
is visible at a time. Tabs are added to the TabPane by using the getTabs()
.
Tabs in a TabPane can be positioned at any of the four sides by specifying the
Side
.
A TabPane has two modes floating or recessed. Applying the styleclass STYLE_CLASS_FLOATING will change the TabPane mode to floating.
The tabs width and height can be set to a specific size by setting the min and max for height and width. TabPane default width will be determined by the largest content width in the TabPane. This is the same for the height. If a different size is desired the width and height of the TabPane can be overridden by setting the min, pref and max size.
When the number of tabs do not fit the TabPane a menu button will appear on the right. The menu button is used to select the tabs that are currently not visible.
Example:
TabPane tabPane = new TabPane();
Tab tab = new Tab();
tab.setText("new tab");
tab.setContent(new Rectangle(100, 50, Color.LIGHTSTEELBLUE));
tabPane.getTabs().add(tab);
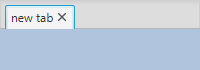
- Since:
- JavaFX 2.0
- See Also:
Tab
-
Property Summary
Properties Type Property Description BooleanProperty
rotateGraphic
The rotateGraphic state of the tabs in the TabPane.ObjectProperty<SingleSelectionModel<Tab>>
selectionModel
The selection model used for selecting tabs.ObjectProperty<Side>
side
The position of the tabs in the TabPane.ObjectProperty<TabPane.TabClosingPolicy>
tabClosingPolicy
The closing policy for the tabs.ObjectProperty<TabPane.TabDragPolicy>
tabDragPolicy
The drag policy for the tabs.DoubleProperty
tabMaxHeight
The maximum height of the tabs in the TabPane.DoubleProperty
tabMaxWidth
The maximum width of the tabs in the TabPane.DoubleProperty
tabMinHeight
The minimum height of the tab.DoubleProperty
tabMinWidth
The minimum width of the tabs in the TabPane.Properties inherited from class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties inherited from class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Nested Class Summary
Nested Classes Modifier and Type Class Description static class
TabPane.TabClosingPolicy
This specifies how the TabPane handles tab closing from an end-users perspective.static class
TabPane.TabDragPolicy
This enum specifies drag policies for tabs in a TabPane. -
Field Summary
Fields Modifier and Type Field Description static String
STYLE_CLASS_FLOATING
TabPane mode will be changed to floating allowing the TabPane to be placed alongside other control. -
Constructor Summary
-
Method Summary
Modifier and Type Method Description protected Skin<?>
createDefaultSkin()
Create a new instance of the default skin for this control.static List<CssMetaData<? extends Styleable,?>>
getClassCssMetaData()
List<CssMetaData<? extends Styleable,?>>
getControlCssMetaData()
SingleSelectionModel<Tab>
getSelectionModel()
Gets the model used for tab selection.Side
getSide()
The current position of the tabs in the TabPane.TabPane.TabClosingPolicy
getTabClosingPolicy()
The closing policy for the tabs.TabPane.TabDragPolicy
getTabDragPolicy()
Gets the value of the property tabDragPolicy.double
getTabMaxHeight()
The maximum height of the tabs in the TabPane.double
getTabMaxWidth()
The maximum width of the tabs in the TabPane.double
getTabMinHeight()
The minimum height of the tabs in the TabPane.double
getTabMinWidth()
The minimum width of the tabs in the TabPane.ObservableList<Tab>
getTabs()
The tabs to display in this TabPane.boolean
isRotateGraphic()
Returnstrue
if the graphic inside a Tab is rotated.Node
lookup(String selector)
Finds thisNode
, or the first sub-node, based on the given CSS selector.Set<Node>
lookupAll(String selector)
Finds allNode
s, including this one and any children, which match the given CSS selector.BooleanProperty
rotateGraphicProperty()
The rotateGraphic state of the tabs in the TabPane.ObjectProperty<SingleSelectionModel<Tab>>
selectionModelProperty()
The selection model used for selecting tabs.void
setRotateGraphic(boolean value)
Specifies whether the graphic inside a Tab is rotated or not, such that it is always upright, or rotated in the same way as the Tab text is.void
setSelectionModel(SingleSelectionModel<Tab> value)
Sets the model used for tab selection.void
setSide(Side value)
The position to place the tabs in this TabPane.void
setTabClosingPolicy(TabPane.TabClosingPolicy value)
Specifies how the TabPane handles tab closing from an end-users perspective.void
setTabDragPolicy(TabPane.TabDragPolicy value)
Sets the value of the property tabDragPolicy.void
setTabMaxHeight(double value)
Sets the value of the property tabMaxHeight.void
setTabMaxWidth(double value)
Sets the value of the property tabMaxWidth.void
setTabMinHeight(double value)
The minimum height of the tabs in the TabPane.void
setTabMinWidth(double value)
The minimum width of the tabs in the TabPane.ObjectProperty<Side>
sideProperty()
The position of the tabs in the TabPane.ObjectProperty<TabPane.TabClosingPolicy>
tabClosingPolicyProperty()
The closing policy for the tabs.ObjectProperty<TabPane.TabDragPolicy>
tabDragPolicyProperty()
The drag policy for the tabs.DoubleProperty
tabMaxHeightProperty()
The maximum height of the tabs in the TabPane.DoubleProperty
tabMaxWidthProperty()
The maximum width of the tabs in the TabPane.DoubleProperty
tabMinHeightProperty()
The minimum height of the tab.DoubleProperty
tabMinWidthProperty()
The minimum width of the tabs in the TabPane.Methods inherited from class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, computePrefHeight, computePrefWidth, contextMenuProperty, executeAccessibleAction, getBaselineOffset, getContextMenu, getCssMetaData, getInitialFocusTraversable, getSkin, getTooltip, isResizable, layoutChildren, queryAccessibleAttribute, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods inherited from class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods inherited from class javafx.scene.Parent
getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods inherited from class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, fireEvent, focusedProperty, focusTraversableProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
-
Property Details
-
selectionModel
The selection model used for selecting tabs. -
side
The position of the tabs in the TabPane.- See Also:
getSide()
,setSide(Side)
-
tabClosingPolicy
The closing policy for the tabs. -
rotateGraphic
The rotateGraphic state of the tabs in the TabPane.- See Also:
isRotateGraphic()
,setRotateGraphic(boolean)
-
tabMinWidth
The minimum width of the tabs in the TabPane.- See Also:
getTabMinWidth()
,setTabMinWidth(double)
-
tabMaxWidth
The maximum width of the tabs in the TabPane.- Returns:
- the maximum width property
-
tabMinHeight
The minimum height of the tab.- See Also:
getTabMinHeight()
,setTabMinHeight(double)
-
tabMaxHeight
The maximum height of the tabs in the TabPane.
- Returns:
- the maximum height of the tabs
-
tabDragPolicy
The drag policy for the tabs. The policy can be changed dynamically.- Default value:
- TabDragPolicy.FIXED
- Since:
- 10
- See Also:
getTabDragPolicy()
,setTabDragPolicy(TabPane.TabDragPolicy)
-
-
Field Details
-
STYLE_CLASS_FLOATING
TabPane mode will be changed to floating allowing the TabPane to be placed alongside other control.- See Also:
- Constant Field Values
-
-
Constructor Details
-
Method Details
-
getTabs
The tabs to display in this TabPane. Changing this ObservableList will immediately result in the TabPane updating to display the new contents of this ObservableList.
If the tabs ObservableList changes, the selected tab will remain the previously selected tab, if it remains within this ObservableList. If the previously selected tab is no longer in the tabs ObservableList, the selected tab will become the first tab in the ObservableList.
- Returns:
- the list of tabs
-
setSelectionModel
Sets the model used for tab selection. By changing the model you can alter how the tabs are selected and which tabs are first or last.
- Parameters:
value
- the selection model
-
getSelectionModel
Gets the model used for tab selection.
- Returns:
- the model used for tab selection
-
selectionModelProperty
The selection model used for selecting tabs. -
setSide
The position to place the tabs in this TabPane. Whenever this changes the TabPane will immediately update the location of the tabs to reflect this.
- Parameters:
value
- the side
-
getSide
The current position of the tabs in the TabPane. The default position for the tabs is Side.Top.- Returns:
- The current position of the tabs in the TabPane.
-
sideProperty
The position of the tabs in the TabPane.- See Also:
getSide()
,setSide(Side)
-
setTabClosingPolicy
Specifies how the TabPane handles tab closing from an end-users perspective. The options are:
- TabClosingPolicy.UNAVAILABLE: Tabs can not be closed by the user
- TabClosingPolicy.SELECTED_TAB: Only the currently selected tab will have the option to be closed, with a graphic next to the tab text being shown. The graphic will disappear when a tab is no longer selected.
- TabClosingPolicy.ALL_TABS: All tabs will have the option to be closed.
Refer to the
The default closing policy is TabClosingPolicy.SELECTED_TABTabPane.TabClosingPolicy
enumeration for further details.- Parameters:
value
- the closing policy
-
getTabClosingPolicy
The closing policy for the tabs.- Returns:
- The closing policy for the tabs.
-
tabClosingPolicyProperty
The closing policy for the tabs. -
setRotateGraphic
public final void setRotateGraphic(boolean value)Specifies whether the graphic inside a Tab is rotated or not, such that it is always upright, or rotated in the same way as the Tab text is.
By default rotateGraphic is set to false, to represent the fact that the graphic isn't rotated, resulting in it always appearing upright. If rotateGraphic is set to
true
, the graphic will rotate such that it rotates with the tab text.- Parameters:
value
- a flag indicating whether to rotate the graphic
-
isRotateGraphic
public final boolean isRotateGraphic()Returnstrue
if the graphic inside a Tab is rotated. The default isfalse
- Returns:
- the rotatedGraphic state.
-
rotateGraphicProperty
The rotateGraphic state of the tabs in the TabPane.- See Also:
isRotateGraphic()
,setRotateGraphic(boolean)
-
setTabMinWidth
public final void setTabMinWidth(double value)The minimum width of the tabs in the TabPane. This can be used to limit the length of text in tabs to prevent truncation. Setting the min equal to the max will fix the width of the tab. By default the min equals to the max. This value can also be set via CSS using
-fx-tab-min-width
- Parameters:
value
- the minimum width of the tabs
-
getTabMinWidth
public final double getTabMinWidth()The minimum width of the tabs in the TabPane.- Returns:
- The minimum width of the tabs
-
tabMinWidthProperty
The minimum width of the tabs in the TabPane.- See Also:
getTabMinWidth()
,setTabMinWidth(double)
-
setTabMaxWidth
public final void setTabMaxWidth(double value)Sets the value of the property tabMaxWidth.- Property description:
Specifies the maximum width of a tab. This can be used to limit the length of text in tabs. If the tab text is longer than the maximum width the text will be truncated. Setting the max equal to the min will fix the width of the tab. By default the min equals to the max This value can also be set via CSS using
-fx-tab-max-width
.
-
getTabMaxWidth
public final double getTabMaxWidth()The maximum width of the tabs in the TabPane.- Returns:
- The maximum width of the tabs
-
tabMaxWidthProperty
The maximum width of the tabs in the TabPane.- Returns:
- the maximum width property
-
setTabMinHeight
public final void setTabMinHeight(double value)The minimum height of the tabs in the TabPane. This can be used to limit the height in tabs. Setting the min equal to the max will fix the height of the tab. By default the min equals to the max. This value can also be set via CSS using
-fx-tab-min-height
- Parameters:
value
- the minimum height of the tabs
-
getTabMinHeight
public final double getTabMinHeight()The minimum height of the tabs in the TabPane.- Returns:
- the minimum height of the tabs
-
tabMinHeightProperty
The minimum height of the tab.- See Also:
getTabMinHeight()
,setTabMinHeight(double)
-
setTabMaxHeight
public final void setTabMaxHeight(double value)Sets the value of the property tabMaxHeight.- Property description:
The maximum height if the tabs in the TabPane. This can be used to limit the height in tabs. Setting the max equal to the min will fix the height of the tab. By default the min equals to the max. This value can also be set via CSS using -fx-tab-max-height
-
getTabMaxHeight
public final double getTabMaxHeight()The maximum height of the tabs in the TabPane.- Returns:
- The maximum height of the tabs
-
tabMaxHeightProperty
The maximum height of the tabs in the TabPane.
- Returns:
- the maximum height of the tabs
-
createDefaultSkin
Create a new instance of the default skin for this control. This is called to create a skin for the control if no skin is provided via CSS-fx-skin
or set explicitly in a sub-class withsetSkin(...)
.- Overrides:
createDefaultSkin
in classControl
- Returns:
- new instance of default skin for this control. If null then the control will have no skin unless one is provided by css.
-
lookup
Finds thisNode
, or the first sub-node, based on the given CSS selector. If this node is aParent
, then this function will traverse down into the branch until it finds a match. If more than one sub-node matches the specified selector, this function returns the first of them.For example, if a Node is given the id of "myId", then the lookup method can be used to find this node as follows:
scene.lookup("#myId");
. -
lookupAll
Finds allNode
s, including this one and any children, which match the given CSS selector. If no matches are found, an empty unmodifiable set is returned. The set is explicitly unordered. -
getClassCssMetaData
- Returns:
- The CssMetaData associated with this class, which may include the CssMetaData of its superclasses.
- Since:
- JavaFX 8.0
-
getControlCssMetaData
- Overrides:
getControlCssMetaData
in classControl
- Returns:
- unmodifiable list of the controls css styleable properties
- Since:
- JavaFX 8.0
-
tabDragPolicyProperty
The drag policy for the tabs. The policy can be changed dynamically.- Default value:
- TabDragPolicy.FIXED
- Since:
- 10
- See Also:
getTabDragPolicy()
,setTabDragPolicy(TabPane.TabDragPolicy)
-
setTabDragPolicy
Sets the value of the property tabDragPolicy.- Property description:
- The drag policy for the tabs. The policy can be changed dynamically.
- Default value:
- TabDragPolicy.FIXED
- Since:
- 10
-
getTabDragPolicy
Gets the value of the property tabDragPolicy.- Property description:
- The drag policy for the tabs. The policy can be changed dynamically.
- Default value:
- TabDragPolicy.FIXED
- Since:
- 10
-