- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
@DefaultProperty("content") public class ScrollPane extends Control
The ScrollPane allows the application to set the current, minimum, and
maximum values for positioning the contents in the horizontal and
vertical directions. These values are mapped proportionally onto the
layoutBounds
of the contained node.
ScrollPane layout calculations are based on the layoutBounds rather than the boundsInParent (visual bounds) of the scroll node. If an application wants the scrolling to be based on the visual bounds of the node (for scaled content etc.), it needs to wrap the scroll node in a Group.
ScrollPane sets focusTraversable to false.
This example creates a ScrollPane, which contains a Rectangle:
Rectangle rect = new Rectangle(200, 200, Color.RED);
ScrollPane s1 = new ScrollPane();
s1.setPrefSize(120, 120);
s1.setContent(rect);
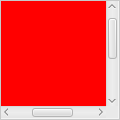
- Since:
- JavaFX 2.0
-
Property Summary
Properties Type Property Description ObjectProperty<Node>
content
The node used as the content of this ScrollPane.BooleanProperty
fitToHeight
If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport.BooleanProperty
fitToWidth
If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport.ObjectProperty<ScrollPane.ScrollBarPolicy>
hbarPolicy
Specifies the policy for showing the horizontal scroll bar.DoubleProperty
hmax
The maximum allowablehvalue
for this ScrollPane.DoubleProperty
hmin
The minimum allowablehvalue
for this ScrollPane.DoubleProperty
hvalue
The current horizontal scroll position of the ScrollPane.DoubleProperty
minViewportHeight
Specify the minimum height of the ScrollPane Viewport.DoubleProperty
minViewportWidth
Specify the minimum width of the ScrollPane Viewport.BooleanProperty
pannable
Specifies whether the user should be able to pan the viewport by using the mouse.DoubleProperty
prefViewportHeight
Specify the preferred height of the ScrollPane Viewport.DoubleProperty
prefViewportWidth
Specify the preferred width of the ScrollPane Viewport.ObjectProperty<ScrollPane.ScrollBarPolicy>
vbarPolicy
Specifies the policy for showing the vertical scroll bar.ObjectProperty<Bounds>
viewportBounds
The actual Bounds of the ScrollPane Viewport.DoubleProperty
vmax
The maximum allowablevvalue
for this ScrollPane.DoubleProperty
vmin
The minimum allowablevvalue
for this ScrollPane.DoubleProperty
vvalue
The current vertical scroll position of the ScrollPane.Properties inherited from class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties inherited from class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Nested Class Summary
Nested Classes Modifier and Type Class Description static class
ScrollPane.ScrollBarPolicy
An enumeration denoting the policy to be used by a scrollable Control in deciding whether to show a scroll bar. -
Field Summary
-
Constructor Summary
Constructors Constructor Description ScrollPane()
Creates a new ScrollPane.ScrollPane(Node content)
Creates a new ScrollPane. -
Method Summary
Modifier and Type Method Description ObjectProperty<Node>
contentProperty()
The node used as the content of this ScrollPane.protected Skin<?>
createDefaultSkin()
Create a new instance of the default skin for this control.BooleanProperty
fitToHeightProperty()
If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport.BooleanProperty
fitToWidthProperty()
If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport.static List<CssMetaData<? extends Styleable,?>>
getClassCssMetaData()
Node
getContent()
Gets the value of the property content.List<CssMetaData<? extends Styleable,?>>
getControlCssMetaData()
ScrollPane.ScrollBarPolicy
getHbarPolicy()
Gets the value of the property hbarPolicy.double
getHmax()
Gets the value of the property hmax.double
getHmin()
Gets the value of the property hmin.double
getHvalue()
Gets the value of the property hvalue.protected Boolean
getInitialFocusTraversable()
Returns the initial focus traversable state of this control, for use by the JavaFX CSS engine to correctly set its initial value.double
getMinViewportHeight()
Gets the value of the property minViewportHeight.double
getMinViewportWidth()
Gets the value of the property minViewportWidth.double
getPrefViewportHeight()
Gets the value of the property prefViewportHeight.double
getPrefViewportWidth()
Gets the value of the property prefViewportWidth.ScrollPane.ScrollBarPolicy
getVbarPolicy()
Gets the value of the property vbarPolicy.Bounds
getViewportBounds()
Gets the value of the property viewportBounds.double
getVmax()
Gets the value of the property vmax.double
getVmin()
Gets the value of the property vmin.double
getVvalue()
Gets the value of the property vvalue.ObjectProperty<ScrollPane.ScrollBarPolicy>
hbarPolicyProperty()
Specifies the policy for showing the horizontal scroll bar.DoubleProperty
hmaxProperty()
The maximum allowablehvalue
for this ScrollPane.DoubleProperty
hminProperty()
The minimum allowablehvalue
for this ScrollPane.DoubleProperty
hvalueProperty()
The current horizontal scroll position of the ScrollPane.boolean
isFitToHeight()
Gets the value of the property fitToHeight.boolean
isFitToWidth()
Gets the value of the property fitToWidth.boolean
isPannable()
Gets the value of the property pannable.DoubleProperty
minViewportHeightProperty()
Specify the minimum height of the ScrollPane Viewport.DoubleProperty
minViewportWidthProperty()
Specify the minimum width of the ScrollPane Viewport.BooleanProperty
pannableProperty()
Specifies whether the user should be able to pan the viewport by using the mouse.DoubleProperty
prefViewportHeightProperty()
Specify the preferred height of the ScrollPane Viewport.DoubleProperty
prefViewportWidthProperty()
Specify the preferred width of the ScrollPane Viewport.Object
queryAccessibleAttribute(AccessibleAttribute attribute, Object... parameters)
This method is called by the assistive technology to request the value for an attribute.void
setContent(Node value)
Sets the value of the property content.void
setFitToHeight(boolean value)
Sets the value of the property fitToHeight.void
setFitToWidth(boolean value)
Sets the value of the property fitToWidth.void
setHbarPolicy(ScrollPane.ScrollBarPolicy value)
Sets the value of the property hbarPolicy.void
setHmax(double value)
Sets the value of the property hmax.void
setHmin(double value)
Sets the value of the property hmin.void
setHvalue(double value)
Sets the value of the property hvalue.void
setMinViewportHeight(double value)
Sets the value of the property minViewportHeight.void
setMinViewportWidth(double value)
Sets the value of the property minViewportWidth.void
setPannable(boolean value)
Sets the value of the property pannable.void
setPrefViewportHeight(double value)
Sets the value of the property prefViewportHeight.void
setPrefViewportWidth(double value)
Sets the value of the property prefViewportWidth.void
setVbarPolicy(ScrollPane.ScrollBarPolicy value)
Sets the value of the property vbarPolicy.void
setViewportBounds(Bounds value)
Sets the value of the property viewportBounds.void
setVmax(double value)
Sets the value of the property vmax.void
setVmin(double value)
Sets the value of the property vmin.void
setVvalue(double value)
Sets the value of the property vvalue.ObjectProperty<ScrollPane.ScrollBarPolicy>
vbarPolicyProperty()
Specifies the policy for showing the vertical scroll bar.ObjectProperty<Bounds>
viewportBoundsProperty()
The actual Bounds of the ScrollPane Viewport.DoubleProperty
vmaxProperty()
The maximum allowablevvalue
for this ScrollPane.DoubleProperty
vminProperty()
The minimum allowablevvalue
for this ScrollPane.DoubleProperty
vvalueProperty()
The current vertical scroll position of the ScrollPane.Methods inherited from class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, computePrefHeight, computePrefWidth, contextMenuProperty, executeAccessibleAction, getBaselineOffset, getContextMenu, getCssMetaData, getSkin, getTooltip, isResizable, layoutChildren, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods inherited from class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods inherited from class javafx.scene.Parent
getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, lookup, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods inherited from class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, fireEvent, focusedProperty, focusTraversableProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
-
Property Details
-
hbarPolicy
Specifies the policy for showing the horizontal scroll bar. -
vbarPolicy
Specifies the policy for showing the vertical scroll bar. -
content
The node used as the content of this ScrollPane.- See Also:
getContent()
,setContent(Node)
-
hvalue
The current horizontal scroll position of the ScrollPane. This value may be set by the application to scroll the view programatically. The ScrollPane will update this value whenever the viewport is scrolled or panned by the user. This value must always be within the range ofhmin
tohmax
. Whenhvalue
equalshmin
, the contained node is positioned so that its layoutBoundsminX
is visible. Whenhvalue
equalshmax
, the contained node is positioned so that its layoutBoundsmaxX
is visible. Whenhvalue
is betweenhmin
andhmax
, the contained node is positioned proportionally between layoutBoundsminX
and layoutBoundsmaxX
.- See Also:
getHvalue()
,setHvalue(double)
-
vvalue
The current vertical scroll position of the ScrollPane. This value may be set by the application to scroll the view programatically. The ScrollPane will update this value whenever the viewport is scrolled or panned by the user. This value must always be within the range ofvmin
tovmax
. Whenvvalue
equalsvmin
, the contained node is positioned so that its layoutBoundsminY
is visible. Whenvvalue
equalsvmax
, the contained node is positioned so that its layoutBoundsmaxY
is visible. Whenvvalue
is betweenvmin
andvmax
, the contained node is positioned proportionally between layoutBoundsminY
and layoutBoundsmaxY
.- See Also:
getVvalue()
,setVvalue(double)
-
hmin
The minimum allowablehvalue
for this ScrollPane. Default value is 0.- See Also:
getHmin()
,setHmin(double)
-
vmin
The minimum allowablevvalue
for this ScrollPane. Default value is 0.- See Also:
getVmin()
,setVmin(double)
-
hmax
The maximum allowablehvalue
for this ScrollPane. Default value is 1.- See Also:
getHmax()
,setHmax(double)
-
vmax
The maximum allowablevvalue
for this ScrollPane. Default value is 1.- See Also:
getVmax()
,setVmax(double)
-
fitToWidth
If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.- See Also:
isFitToWidth()
,setFitToWidth(boolean)
-
fitToHeight
If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.- See Also:
isFitToHeight()
,setFitToHeight(boolean)
-
pannable
Specifies whether the user should be able to pan the viewport by using the mouse. If mouse events reach the ScrollPane (that is, if mouse events are not blocked by the contained node or one of its children) thenpannable
is consulted to determine if the events should be used for panning.- See Also:
isPannable()
,setPannable(boolean)
-
prefViewportWidth
Specify the preferred width of the ScrollPane Viewport. This is the width that will be available to the content node. The overall width of the ScrollPane is the ViewportWidth + padding -
prefViewportHeight
Specify the preferred height of the ScrollPane Viewport. This is the height that will be available to the content node. The overall height of the ScrollPane is the ViewportHeight + padding -
minViewportWidth
Specify the minimum width of the ScrollPane Viewport. This is the width that will be available to the content node.- Since:
- JavaFX 8u40
- See Also:
getMinViewportWidth()
,setMinViewportWidth(double)
-
minViewportHeight
Specify the minimum height of the ScrollPane Viewport. This is the height that will be available to the content node.- Since:
- JavaFX 8u40
- See Also:
getMinViewportHeight()
,setMinViewportHeight(double)
-
viewportBounds
The actual Bounds of the ScrollPane Viewport. This is the Bounds of the content node.- See Also:
getViewportBounds()
,setViewportBounds(Bounds)
-
-
Constructor Details
-
ScrollPane
public ScrollPane()Creates a new ScrollPane. -
ScrollPane
Creates a new ScrollPane.- Parameters:
content
- the initial content for the ScrollPane- Since:
- JavaFX 8.0
-
-
Method Details
-
setHbarPolicy
Sets the value of the property hbarPolicy.- Property description:
- Specifies the policy for showing the horizontal scroll bar.
-
getHbarPolicy
Gets the value of the property hbarPolicy.- Property description:
- Specifies the policy for showing the horizontal scroll bar.
-
hbarPolicyProperty
Specifies the policy for showing the horizontal scroll bar. -
setVbarPolicy
Sets the value of the property vbarPolicy.- Property description:
- Specifies the policy for showing the vertical scroll bar.
-
getVbarPolicy
Gets the value of the property vbarPolicy.- Property description:
- Specifies the policy for showing the vertical scroll bar.
-
vbarPolicyProperty
Specifies the policy for showing the vertical scroll bar. -
setContent
Sets the value of the property content.- Property description:
- The node used as the content of this ScrollPane.
-
getContent
Gets the value of the property content.- Property description:
- The node used as the content of this ScrollPane.
-
contentProperty
The node used as the content of this ScrollPane.- See Also:
getContent()
,setContent(Node)
-
setHvalue
public final void setHvalue(double value)Sets the value of the property hvalue.- Property description:
- The current horizontal scroll position of the ScrollPane. This value
may be set by the application to scroll the view programatically.
The ScrollPane will update this value whenever the viewport is
scrolled or panned by the user. This value must always be within
the range of
hmin
tohmax
. Whenhvalue
equalshmin
, the contained node is positioned so that its layoutBoundsminX
is visible. Whenhvalue
equalshmax
, the contained node is positioned so that its layoutBoundsmaxX
is visible. Whenhvalue
is betweenhmin
andhmax
, the contained node is positioned proportionally between layoutBoundsminX
and layoutBoundsmaxX
.
-
getHvalue
public final double getHvalue()Gets the value of the property hvalue.- Property description:
- The current horizontal scroll position of the ScrollPane. This value
may be set by the application to scroll the view programatically.
The ScrollPane will update this value whenever the viewport is
scrolled or panned by the user. This value must always be within
the range of
hmin
tohmax
. Whenhvalue
equalshmin
, the contained node is positioned so that its layoutBoundsminX
is visible. Whenhvalue
equalshmax
, the contained node is positioned so that its layoutBoundsmaxX
is visible. Whenhvalue
is betweenhmin
andhmax
, the contained node is positioned proportionally between layoutBoundsminX
and layoutBoundsmaxX
.
-
hvalueProperty
The current horizontal scroll position of the ScrollPane. This value may be set by the application to scroll the view programatically. The ScrollPane will update this value whenever the viewport is scrolled or panned by the user. This value must always be within the range ofhmin
tohmax
. Whenhvalue
equalshmin
, the contained node is positioned so that its layoutBoundsminX
is visible. Whenhvalue
equalshmax
, the contained node is positioned so that its layoutBoundsmaxX
is visible. Whenhvalue
is betweenhmin
andhmax
, the contained node is positioned proportionally between layoutBoundsminX
and layoutBoundsmaxX
.- See Also:
getHvalue()
,setHvalue(double)
-
setVvalue
public final void setVvalue(double value)Sets the value of the property vvalue.- Property description:
- The current vertical scroll position of the ScrollPane. This value
may be set by the application to scroll the view programatically.
The ScrollPane will update this value whenever the viewport is
scrolled or panned by the user. This value must always be within
the range of
vmin
tovmax
. Whenvvalue
equalsvmin
, the contained node is positioned so that its layoutBoundsminY
is visible. Whenvvalue
equalsvmax
, the contained node is positioned so that its layoutBoundsmaxY
is visible. Whenvvalue
is betweenvmin
andvmax
, the contained node is positioned proportionally between layoutBoundsminY
and layoutBoundsmaxY
.
-
getVvalue
public final double getVvalue()Gets the value of the property vvalue.- Property description:
- The current vertical scroll position of the ScrollPane. This value
may be set by the application to scroll the view programatically.
The ScrollPane will update this value whenever the viewport is
scrolled or panned by the user. This value must always be within
the range of
vmin
tovmax
. Whenvvalue
equalsvmin
, the contained node is positioned so that its layoutBoundsminY
is visible. Whenvvalue
equalsvmax
, the contained node is positioned so that its layoutBoundsmaxY
is visible. Whenvvalue
is betweenvmin
andvmax
, the contained node is positioned proportionally between layoutBoundsminY
and layoutBoundsmaxY
.
-
vvalueProperty
The current vertical scroll position of the ScrollPane. This value may be set by the application to scroll the view programatically. The ScrollPane will update this value whenever the viewport is scrolled or panned by the user. This value must always be within the range ofvmin
tovmax
. Whenvvalue
equalsvmin
, the contained node is positioned so that its layoutBoundsminY
is visible. Whenvvalue
equalsvmax
, the contained node is positioned so that its layoutBoundsmaxY
is visible. Whenvvalue
is betweenvmin
andvmax
, the contained node is positioned proportionally between layoutBoundsminY
and layoutBoundsmaxY
.- See Also:
getVvalue()
,setVvalue(double)
-
setHmin
public final void setHmin(double value)Sets the value of the property hmin.- Property description:
- The minimum allowable
hvalue
for this ScrollPane. Default value is 0.
-
getHmin
public final double getHmin()Gets the value of the property hmin.- Property description:
- The minimum allowable
hvalue
for this ScrollPane. Default value is 0.
-
hminProperty
The minimum allowablehvalue
for this ScrollPane. Default value is 0.- See Also:
getHmin()
,setHmin(double)
-
setVmin
public final void setVmin(double value)Sets the value of the property vmin.- Property description:
- The minimum allowable
vvalue
for this ScrollPane. Default value is 0.
-
getVmin
public final double getVmin()Gets the value of the property vmin.- Property description:
- The minimum allowable
vvalue
for this ScrollPane. Default value is 0.
-
vminProperty
The minimum allowablevvalue
for this ScrollPane. Default value is 0.- See Also:
getVmin()
,setVmin(double)
-
setHmax
public final void setHmax(double value)Sets the value of the property hmax.- Property description:
- The maximum allowable
hvalue
for this ScrollPane. Default value is 1.
-
getHmax
public final double getHmax()Gets the value of the property hmax.- Property description:
- The maximum allowable
hvalue
for this ScrollPane. Default value is 1.
-
hmaxProperty
The maximum allowablehvalue
for this ScrollPane. Default value is 1.- See Also:
getHmax()
,setHmax(double)
-
setVmax
public final void setVmax(double value)Sets the value of the property vmax.- Property description:
- The maximum allowable
vvalue
for this ScrollPane. Default value is 1.
-
getVmax
public final double getVmax()Gets the value of the property vmax.- Property description:
- The maximum allowable
vvalue
for this ScrollPane. Default value is 1.
-
vmaxProperty
The maximum allowablevvalue
for this ScrollPane. Default value is 1.- See Also:
getVmax()
,setVmax(double)
-
setFitToWidth
public final void setFitToWidth(boolean value)Sets the value of the property fitToWidth.- Property description:
- If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.
-
isFitToWidth
public final boolean isFitToWidth()Gets the value of the property fitToWidth.- Property description:
- If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.
-
fitToWidthProperty
If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.- See Also:
isFitToWidth()
,setFitToWidth(boolean)
-
setFitToHeight
public final void setFitToHeight(boolean value)Sets the value of the property fitToHeight.- Property description:
- If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.
-
isFitToHeight
public final boolean isFitToHeight()Gets the value of the property fitToHeight.- Property description:
- If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.
-
fitToHeightProperty
If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.- See Also:
isFitToHeight()
,setFitToHeight(boolean)
-
setPannable
public final void setPannable(boolean value)Sets the value of the property pannable.- Property description:
- Specifies whether the user should be able to pan the viewport by using
the mouse. If mouse events reach the ScrollPane (that is, if mouse
events are not blocked by the contained node or one of its children)
then
pannable
is consulted to determine if the events should be used for panning.
-
isPannable
public final boolean isPannable()Gets the value of the property pannable.- Property description:
- Specifies whether the user should be able to pan the viewport by using
the mouse. If mouse events reach the ScrollPane (that is, if mouse
events are not blocked by the contained node or one of its children)
then
pannable
is consulted to determine if the events should be used for panning.
-
pannableProperty
Specifies whether the user should be able to pan the viewport by using the mouse. If mouse events reach the ScrollPane (that is, if mouse events are not blocked by the contained node or one of its children) thenpannable
is consulted to determine if the events should be used for panning.- See Also:
isPannable()
,setPannable(boolean)
-
setPrefViewportWidth
public final void setPrefViewportWidth(double value)Sets the value of the property prefViewportWidth.- Property description:
- Specify the preferred width of the ScrollPane Viewport. This is the width that will be available to the content node. The overall width of the ScrollPane is the ViewportWidth + padding
-
getPrefViewportWidth
public final double getPrefViewportWidth()Gets the value of the property prefViewportWidth.- Property description:
- Specify the preferred width of the ScrollPane Viewport. This is the width that will be available to the content node. The overall width of the ScrollPane is the ViewportWidth + padding
-
prefViewportWidthProperty
Specify the preferred width of the ScrollPane Viewport. This is the width that will be available to the content node. The overall width of the ScrollPane is the ViewportWidth + padding -
setPrefViewportHeight
public final void setPrefViewportHeight(double value)Sets the value of the property prefViewportHeight.- Property description:
- Specify the preferred height of the ScrollPane Viewport. This is the height that will be available to the content node. The overall height of the ScrollPane is the ViewportHeight + padding
-
getPrefViewportHeight
public final double getPrefViewportHeight()Gets the value of the property prefViewportHeight.- Property description:
- Specify the preferred height of the ScrollPane Viewport. This is the height that will be available to the content node. The overall height of the ScrollPane is the ViewportHeight + padding
-
prefViewportHeightProperty
Specify the preferred height of the ScrollPane Viewport. This is the height that will be available to the content node. The overall height of the ScrollPane is the ViewportHeight + padding -
setMinViewportWidth
public final void setMinViewportWidth(double value)Sets the value of the property minViewportWidth.- Property description:
- Specify the minimum width of the ScrollPane Viewport. This is the width that will be available to the content node.
- Since:
- JavaFX 8u40
-
getMinViewportWidth
public final double getMinViewportWidth()Gets the value of the property minViewportWidth.- Property description:
- Specify the minimum width of the ScrollPane Viewport. This is the width that will be available to the content node.
- Since:
- JavaFX 8u40
-
minViewportWidthProperty
Specify the minimum width of the ScrollPane Viewport. This is the width that will be available to the content node.- Since:
- JavaFX 8u40
- See Also:
getMinViewportWidth()
,setMinViewportWidth(double)
-
setMinViewportHeight
public final void setMinViewportHeight(double value)Sets the value of the property minViewportHeight.- Property description:
- Specify the minimum height of the ScrollPane Viewport. This is the height that will be available to the content node.
- Since:
- JavaFX 8u40
-
getMinViewportHeight
public final double getMinViewportHeight()Gets the value of the property minViewportHeight.- Property description:
- Specify the minimum height of the ScrollPane Viewport. This is the height that will be available to the content node.
- Since:
- JavaFX 8u40
-
minViewportHeightProperty
Specify the minimum height of the ScrollPane Viewport. This is the height that will be available to the content node.- Since:
- JavaFX 8u40
- See Also:
getMinViewportHeight()
,setMinViewportHeight(double)
-
setViewportBounds
Sets the value of the property viewportBounds.- Property description:
- The actual Bounds of the ScrollPane Viewport. This is the Bounds of the content node.
-
getViewportBounds
Gets the value of the property viewportBounds.- Property description:
- The actual Bounds of the ScrollPane Viewport. This is the Bounds of the content node.
-
viewportBoundsProperty
The actual Bounds of the ScrollPane Viewport. This is the Bounds of the content node.- See Also:
getViewportBounds()
,setViewportBounds(Bounds)
-
createDefaultSkin
Create a new instance of the default skin for this control. This is called to create a skin for the control if no skin is provided via CSS-fx-skin
or set explicitly in a sub-class withsetSkin(...)
.- Overrides:
createDefaultSkin
in classControl
- Returns:
- new instance of default skin for this control. If null then the control will have no skin unless one is provided by css.
-
getClassCssMetaData
- Returns:
- The CssMetaData associated with this class, which may include the CssMetaData of its superclasses.
- Since:
- JavaFX 8.0
-
getControlCssMetaData
- Overrides:
getControlCssMetaData
in classControl
- Returns:
- unmodifiable list of the controls css styleable properties
- Since:
- JavaFX 8.0
-
getInitialFocusTraversable
Returns the initial focus traversable state of this control, for use by the JavaFX CSS engine to correctly set its initial value. This method is overridden as by default UI controls have focus traversable set to true, but that is not appropriate for this control.- Overrides:
getInitialFocusTraversable
in classControl
- Returns:
- the initial focus traversable state of this control
- Since:
- 9
-
queryAccessibleAttribute
This method is called by the assistive technology to request the value for an attribute.This method is commonly overridden by subclasses to implement attributes that are required for a specific role.
If a particular attribute is not handled, the superclass implementation must be called.- Overrides:
queryAccessibleAttribute
in classControl
- Parameters:
attribute
- the requested attributeparameters
- optional list of parameters- Returns:
- the value for the requested attribute
- See Also:
AccessibleAttribute
-